Setting up a directory structure and using Application.cfm and OnRequestEnd.cfm files are only part of creating an application. Another large part of the equation is the capability to maintain state. Step 2, "Using Variables," mentioned that HTTP and HTML are stateless, meaning that the web server treats every request it receives as a new request and has no knowledge of anything that has transpired previously. This makes it difficult to keep track of progressive operations, such as adding items to a shopping cart. It would be exasperating (if not a little humorous) if every time you made a new page request the web server emptied your shopping cart to start over again. So far, we have only been able to maintain state by passing variable values via the URL or Form scopes. However, it would be a very tedious chore indeed if we tried to pass values to every page in the site using these methods. Besides, having information in the URL means putting it out there for all to see, and there is a lot of information in our application (such as passwords and data source names) that we do not want to share with the general public. It would be nice to have some way to set the value of a variable once and have it available to any template that required it. It would be kind of a temporary holding area for information, some way for us to say to the server, "Here, hold on to this value for me for a while. I will probably need it again in a few minutes." Well, lucky for us we canby using shared memory scopes. Shared memory scopes use memory on ColdFusion Server as a temporary area to store information. There are three shared memory scopes we can use, depending on what information we want to store and where we want to be able to use that information. These three scopes are the Session, Application, and Server scopes, and they are discussed in the following sections. There is also another scope that can be used to track user state throughout our application, and it does not involve using shared server memory. This scope is the Client scope. We will discuss this scope briefly, but it is not the primary focus of this step. For a full discussion of the Client scope, go to the www.LearnColdFusionMX.com web site. The Session Scope As previously mentioned, a session starts when a new visitor arrives at our site and ends when that visitor logs out (manually ends the session) or the session times out. When a new visitor arrives, ColdFusion Server sets aside a portion of its memory to store variables that relate to that particular visitor, as illustrated in Figure 8.7. Figure 8.7. User sessions in server memory. 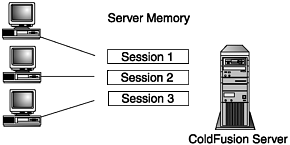 This server memory is an ideal place to temporarily store information about that user as he moves through the site. Variables stored in this temporary memory area are called Session scope variables. These Session scope variables are commonly used to track things like whether a user is logged in or the number of items in his shopping cart. When the user logs out or his session times out, the server reclaims this memory space and wipes away all information stored there. You set and use Session scope variables in a way that's very similar to any other variable. For example, the following line of code would set a variable called Session.UserName equal to the value of "Barry". <CFLOCK SCOPE="SESSION" TIMEOUT="10" TYPE="EXCLUSIVE" > <CFSET Session.UserName="Barry"> </CFLOCK> You are undoubtedly wondering about the <CFLOCK> code used here. Whenever we set or read a shared memory variable, it is best to lock this variable for the time we are accessing it. Locking shared memory variables is covered in more detail in the "Using <CFLOCK>" section later in this step. For the time being, let's just say that it's a really good idea and we need to do it. At any rate, in the preceding code you can see that, aside from this locking business, setting a Session variable is no different than setting any other variable; we simply use the <CFSET> tag. We can use this variable in much the same way that we use any other variable. The following code shows a customized greeting based on a Session variable: <CFOUTPUT> Welcome back <CFLOCK SCOPE="SESSION" TIMEOUT="10" TYPE="READONLY" >#Session.UserName#</CFLOCK> </CFOUTPUT> Again, aside from the locking code, you can see that the use of Session variables is no different than the use of Form or URL scope variables. There is one thing to note for those of you out there who think you can get away with being lazy. You must use the Session scope prefix when using Session scope variables. In this case, using just #UserName# to output the variable isn't going to cut it. If you cast your mind back to Step 2, we discussed a search order that ColdFusion uses to search through variable scopes if you omit the scope prefix from your variable names. Well, the shared memory scopesSession, Application, and Serverare not in that search order, so if you omit the scope prefix, those variables will not be found. Flip back and have a look if don't believe me. Variables stored in the Session scope of a particular user are only available or "visible" to that user. For example, Visitor A would not be able to access or "see" the contents of Visitor B's shopping cart. Each visitor will have his own session scope in memory, and that Session scope is unique for that individual visitor. Some of you out there might be asking, "If we have hundreds of visitors and hundreds of these individual Session scopes, how does ColdFusion Server know which Session scope belongs to which visitor?" I am glad you asked. By default, ColdFusion tracks individuals through the use of browser cookies. A cookie is small text file that the browser writes to that user's hard drive. With Session management enabled, when a visitor arrives at our site, ColdFusion Server checks for the existence of two identity cookies. These cookies store two variables, one called CFID and another called CFToken. CFID is a sequential client identifier, and CFToken is a random-number security token. Together, these two values uniquely identify each site visitor. With every page request, ColdFusion Server checks for the existence of these cookies. If the cookies do not exist, the server will assume that the request is coming from a new visitor and will generate two new cookies for that visitor. The server will also create a new Session scope space in memory and map that user's CFID and CFToken to this new session. When a visitor who already has these CFID and CFToken cookies makes a page request, ColdFusion Server reads these cookies to establish the visitor's identity and maps them to their particular session in memory. All this takes place transparently, and there is nothing that either the visitors or you as a developer need to do. When a visitor's session times out, the cookies expire, and the corresponding session in server memory is wiped away. The only problem with this system is that a visitor might disable the use of cookies in his browser settings, in which case ColdFusion Server will not be able to set the identity cookies on his computer, and he will not get a session set up in memory. You can still use sessions without the use of cookies by passing the user's identity along as a URL variable. However, this becomes a bit more complicated and tedious. For more information on using sessions without cookies, go to the www.LearnColdFusionMX.com web site. If you are interested, you can access the visitor's identity by using the built-in Session variables listed in Table 8.2. Table 8.2. Built-In Session Variables Variable | Description |
---|
Session.CFID | This is the visitor's client ID. It is the same value stored in the CFID cookie on the user's computer. | Session.CFToken | This is the visitor's security token. It is the same value stored in the CFToken cookie on the user's computer. | Session.URLToken | This is a combination of the CFID and CFToken values, and it is used when passing identity information via a URL variable. | The Application Scope It is quite common for a ColdFusion Server to be hosting more that one web site. As previously mentioned, we can logically separate these different applications by using the <CFAPPLICATION> tag and giving each individual application a name. By doing this, not only are we letting all the pages in the web site know that they are part of an application, but by naming our application, we are also setting up a shared memory space for our application as a whole, a place where we can store Application scope variables. Whereas Session scope variables are only available for an individual, Application scope variables can be accessed by anyone using the application. The server in Figure 8.8 is hosting two applications. By using the <CFAPPLICATION> tag and the NAME attribute, we have set up two separate memory areas where we can store Application scope variables. When we set an Application scope variable value for Application 1, that value is available to all users of Application 1 but not the users of Application 2 (and vise versa). This makes the Application scope an ideal place to store general information about the application or information that does not change frequently. For example, if we had several forms in our application that had drop-down menus with standard options like state names, country names, or department titles, we could query our database once and then store the results of the query as an Application variable. By doing this, we would avoid having to connect to the database, run a SQL statement, and retrieve a set of values that is relatively static. When we need the information to build a form, we could just use the values stored in the Application variable instead of querying the database every time. Figure 8.8. Application scope shared memory. 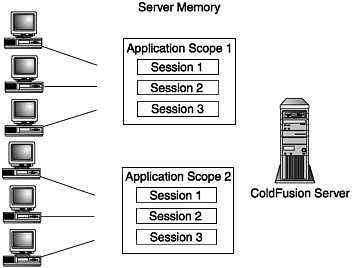 Like Session scope variables, Application scope variables must be enabled in ColdFusion Administrator. (The default setting is enabled.) However, there is no attribute of the <CFAPPLICATION> tag to enable Application scope variables as there is with Session scope variables. The very act of using a <CFAPPLICATION> tag tells ColdFusion Server that you would like to use Application scope variables. In addition, as with Session scope variables, Application scope variables have a lifespan. This lifespan is set in ColdFusion Administrator (refer to Figure 8.5). The default timeout for Application variables is two days. This means that if two days go by and there is not a single page request anywhere in your application, all your Application variables will timeout. You can change the default lifespan by using the optional APPLICATIONTIMEOUT attribute of the <CFAPPLICATION> tag. Like the SESSIONTIMEOUT attribute, you can set the APPLICATIONTIMEOUT value to anything up to the maximum timeout value set in ColdFusion Administrator. We set and use Application scope variables the same way we use Session variables. The following lines of code show how to set and use Application variables: <!--- Set the address for technical contact ---> <CFLOCK SCOPE="SESSION" TIMEOUT="10" TYPE="EXCLUSIVE" > <CFSET Application.WebmasterAddress="hhansen@work.com"> </CFLOCK> <!--- use the variable in another page ---> <CFOUTPUT> If you are experiencing technical problems with this site please contact the <CFLOCK SCOPE="APPLICATION" TIMEOUT="10" TYPE="READONLY"> <A HREF="mailto:#Application.WebmasterAddress#">Webmaster</A> </CFLOCK> </CFOUTPUT> Because Application scope variables also use shared memory on the server, they should be locked in the same way as Session variables. The Application scope comes with one built-in variable, Application.ApplicationName. As you might guess, this will display the name of the application as set in the <CFAPPLICATION> tag. The Server Scope The last of the shared memory scopes is the Server scope. The Server scope is an area of server memory in which we can store variables for the server computer as a whole. Variables stored in the Server scope are available to all users in all applications hosted on that particular server. Using Figure 8.9 as an example, if we were to store a variable in the server scope, that variable would be available to any user visiting either Application 1 or Application 2. For example, we could use a variable called Server.PageCount to keep track of how many page requests have been made to our server by adding the following lines of code to the Application.cfm file for each application hosted on the server. Figure 8.9. Server scope shared memory. 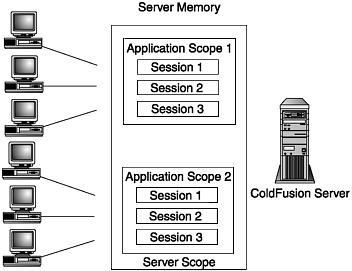 <CFLOCK SCOPE="SERVER" TIMEOUT="10" TYPE="EXCLUSIVE" > <CFPARAM NAME="Server.PageCount" DEFAULT="0"> <CFSET Server.PageCount = Server.PageCount + 1> </CFLOCK> In the preceding code, we first lock the variable. We then use a <CFPARAM> tag to check for the existence of the variable Server.PageCount, and we set its value to zero if it does not already have a value. Then we simply increment the variable value by one. If we include this code in every Application.cfm file on the server, it should run before every page request made to any application. This way, we can keep track of the number of ColdFusion page requests our server has served up. We can then output the code on some type of administration page using the following code: <CFOUTPUT> This server has delivered <CFLOCK SCOPE="SERVER" TIMEOUT="10" TYPE="READONLY"> #Server.PageCount# </CFLOCK> pages </CFOUTPUT> There are some built-in Server scope variables that you can use for administering your server. These variables are listed in Table 8.3. Table 8.3. Built-In Server Scope Variables Variable | Description |
---|
Server.ColdFusion.ProductName | The name of the product (ColdFusion Server). | Server.ColdFusion.Productlevel | The product level: Professional or Enterprise. | Server.ColdFusion.ProductVersion | The product version. ColdFusion MX is version 6.0. | Server.Coldfusion.Expiration | The date on which the server license expires. | Server.ColdFusion.SerialNumber | The serial number for the server installation. | Server.ColdFusion.RootDir | The install directory for ColdFusion, such as C:\CfusionMX. | Server.ColdFusion.SupportedLocales | The various locale settings that this server supports. | Server.OS.Name | The operating system name, such as Windows 2000. | Server.OS.Version | The operating system version number, such as 5.0. | Server.OS.BuildNumber | The operating system build number. | Server.OS.Arch | The processor architecture for the server, such as x86. | Server.OS.AdditionalInformation | Any additional information provided by the operating system. | There is no maximum or default timeout for Server scope variables; they will "live" as long as the server is turned on. Because all shared memory scope variables live in RAM on the server, any power down of the server will result in the loss of all Session, Application, and Server scope variables. The Client Scope There is another scope that can be used to maintain state: the Client scope. Unlike the other scopes previously mentioned, the Client scope does not use shared memory on the server to maintain its information. Client scope variables are stored on disk. We will not discuss the Client scope in depth here because that could take up a whole other chapter. This section is designed as a brief overview to Client scope variables. For more information on Client scope variables, consult the ColdFusion documentation from Macromedia or visit the www.LearnColdFusionMX.com web site. The Client scope is most similar to the Session scope. It is used to track information about a single visitor. The Client scope uses the same two CFID and CFToken cookies as the Session scope when tracking individual visitors. Because Client scope variables are written to disk, however, they can persist over multiple visits or sessions. These variables are commonly used to keep track of visitor preferences, such as color choice or areas of interest. ColdFusion can use three possible methods to store Client scope variables: Registry. Variables will be stored in the Registry of the ColdFusion Server computer. Datasource. Variables will be stored in a data source nominated in ColdFusion Administrator. Cookie. Variables will be stored using cookies on the client's/visitor's computer. To use Client scope variables, they must be enabled using the <CFAPPLICATION> tag. To enable the use of Client variables, you simply set the CLIENTMANAGEMENT attribute to YES. You can also use the CLIENTSTORAGE attribute to specify how you would like to store the Client scope variables. If no CLIENTSTORAGE attribute is set, the setting in ColdFusion Administrator will be used. The default setting is Registry. One limitation of Client scope variables is that they can only store simple data types, such as strings, numbers, and lists. They cannot be used to store arrays, structures, recordsets, or other objects. This becomes important later when we begin to build our shopping cart. When trying to decide which scope to use, it is not a matter of selecting just one. Many applications use a combination of some or all of the scopes previously mentioned. If you have a variable and you are not sure which scope to store it in, ask yourself, "How long does it need to "live," and who needs to see it?" Table 8.4 summarizes the scopes and their uses. Table 8.4. Scope Summary | Client | Session | Application | Server |
---|
Used to track information about: | A single user | A single user | An entire application | An entire server machine | Variables can be accessed by: | A single user | A single user | All users of an application | All users of all applications on that server | Variables can live: | Over multiple sessions for a single user | Over one session for one user | As long as the power stays on and the site stays active | As long as the power stays on | |