In this task, you will use a List control in the form to display the units in which the grocery items are sold, such as pounds or dozens. This data will be dynamically populated from an HTTPService call. You will use an ArrayCollection in the data binding. 1. | Open a web browser and browse to the following URL:
www.flexgrocer.com/units.xml
Notice the structure of the XML. Your goal is to populate a List control in the form with this information.
| 2. | Open DataEntry.mxml. In the existing script block, import the following classes:
mx.collections.ArrayCollection mx.rpc.events.ResultEvent utils.Util You need these classes for the additions you will be making to the DataEntry application in this lesson. The Util class has been custom built for this book.
| | | 3. | Below the import statements, but above the function declaration, add a bindable, private variable named units data typed as ArrayCollection and set it equal to a new ArrayCollection.
[Bindable] private var units:ArrayCollection=new ArrayCollection(); You will be binding an ArrayCollection to the List control. As a best practice, it makes sense to use an ArrayCollection for data binding with a complex data structure because you want it to update when any changes are made to the data structure.
| 4. | Directly below the <mx:Script> block, add an <mx:HTTPService> tag, assign it an id of unitRPC, and specify the url property to http://www.flexgrocer.com/units.xml. Specify the result handler to call unitRPCResult handler and be sure to pass the event object, as follows:
<mx:HTTPService url="http://www.flexgrocer.com/units.xml" result="unitRPCResult(event)"/> You are specifying the URL of the HTTPService to point to the XML you examined in step 1. In the next step, you will write a result handler with the name of unitRPCResult() that will be called when the data has been retrieved. The result event is an event of the HTTPService class that is called when the last byte of data has been retrieved from the remote server. The event will call an ActionScript function when the data has been returned successfully, which enables you to manipulate the returned data.
| | | 5. | Returning to the script block, add a new private function with the name of unitRPCResult(). Be sure to name the parameter event and specify the type as a ResultEvent. Populate the units ArrayCollection with the event.result property from the event object. Remember from examining the XML that the repeating node is unit.
private function unitRPCResult(event:ResultEvent):void{ units=event.result.allUnits.unit; } The event object is created when the result event is broadcast. This object should be strictly typed to the type of event that is occurring; in this case a ResultEvent. All events descend from the Event class in ActionScript. Flex creates one object per event that occurs. The object created contains properties of the standard Event class, but also contains properties unique to the specific type of event that occurred; in this case a ResultEvent object, referred to as event in a function parameter. One of these properties is the result property, which contains all the returned data in whatever format you specified; in this case the default type converts the XML into an ArrayCollection. You have to understand the structure of the retrieved XML to further specify the actual data, in this case allUnits.unit, is appended to event.result. You must "point" to the repeating node in the XML, which is in this case unit.
| 6. | Move to the top of the application and add a creationComplete event that calls the unitRPC HTTPService using the send() method.
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml" layout="absolute" creationComplete="unitRPC.send()"> The object created from the HTTPService class, with the id of unitRPC, must invoke its send() method to actually make the call to the URL. Most of the controls in the grocery store application depend on the data retrieved from the HTTPService. Therefore, you want to be sure that each control has been initialized and is ready for use. So, it makes sense to call the data service on the creationComplete event of the <mx:Application> tag when each control has been drawn, is initialized, and is ready for use.
| 7. | Locate the ProductNameUnit FormItem and change the label to just Unit and remove the direction="horizontal" property assignment. Locate the ComboBox control. Replace the ComboBox control with a List control and delete the TextInput control. Assign the List control an id of unitID and set the rowCount variable to 4. Specify the dataProvider property to the units ArrayCollection and specify the labelField as unitName. Your <mx:List> tag should look as shown here:
<mx:List rowCount="4" dataProvider="{units}" labelField="unitName"/> This will create a List control that will display the units of measurement for the administration tool. You created the units data provider using the ArrayCollection class. From examining the XML, you know that the unitName property is the label you want displayed on the list.
| | | 8. | Save and run the application.
You should see that the List control is populated with the units, as shown here.
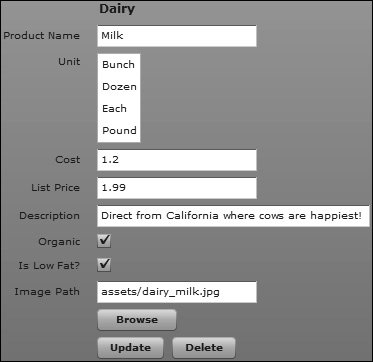 | 9. | Close the file.
You will be working with a different application in the next task.
| |