In the age of the Internet, you may find your programs running in multiple countries. Each country may use a different notation for numbers and dates. For example, some countries use the pound (£) instead of the dollar ($) symbol to indicate currency. The Windows operating system allows the user to set up numeric, date, time, and currency formats specific to their locale. Figure 6.5 shows the Control Panel screen from Windows 2000, which determines the default numeric formats. Figure 6.5. To change the default format for dates and other numbers, use the Regional Options icon in the Windows Control Panel. 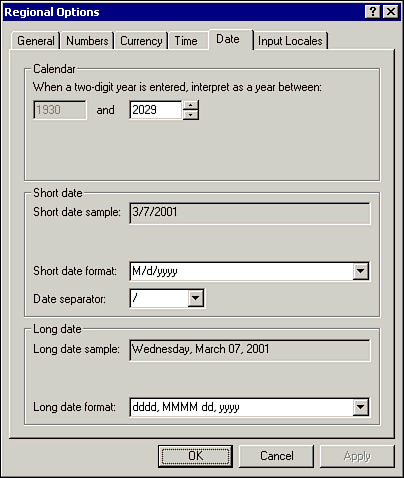 What does this mean to the Visual Basic programmer? There are a couple of ways you can use region (or culture) information: Predefined format strings allow your program to automatically display data in the correct regional format. The System.Globalization namespace provides capabilities to determine information about the formats in a culture. Using Named Formats In earlier sections, we showed how to format dates and numbers using custom strings containing characters representing the desired format of the number. However, it is worth noting that the predefined formats for dates and numbers change depending on the format set in the control panel, as in the following examples: Dim MyDate As Date = #12/10/1972.htm# Debug.WriteLine(Format(MyDate, "d")) Debug.WriteLine(Format(MyDate, "D")) The d and D predefined date formats correspond to the short and long date formats shown in Figure 6.5. If you modify these settings and restart your program, the output format of the preceding lines of code will change. Note The .ToString conversion function for numbers uses the current culture. Using Formats from Other Cultures The System.Globalization namespace contains information about various cultures and the formats they use. Up to this point, we have demonstrated formatting by using the Format function, which always uses the current culture. However, the ToString method of the numeric and date data types can be used with any culture installed on your machine. You simply pass the format string and an instance of the CultureInfo class and the number is formatted appropriately, as in the following example: Dim TestNumber As Integer = 123456 Dim strMessage As String Dim RegInfo As CultureInfo For Each RegInfo In CultureInfo.GetCultures(CultureTypes.InstalledWin32Cultures) strMessage = "The " & RegInfo.NativeName & " number format is" strMessage &= TestNumber.ToString("n", RegInfo.NumberFormat) ListBox1.Items.Add(strMessage) Next The code sample demonstrates using the standard predefined number format (n) of each culture. Figure 6.6 shows the different formats displayed by the previous code. Figure 6.6. The CultureInfo class for a culture contains formatting information for the country or region. 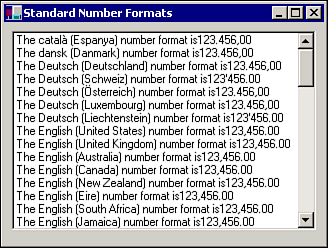 Note The Application.CurrentCulture property contains information about the current culture settings. If you do not specify a format provider, the ToString method uses the current culture settings. Exercise: Parsing Strings In this chapter, you have learned about several functions to manipulate strings. You have also seen how arrays can be used to store multiple variables together. In this section we will combine your knowledge of arrays and strings and introduce two useful methods, Split and Join. The Split function parses a string into array elements, and the Join function combines array elements into a string. To begin this exercise, start a new Windows application project in Visual Studio .NET. Using Split to Separate a String If you are exchanging data with another company, you may be receiving a file delimited by commas, spaces, or tabs. For example, I use a program to pull stock information off a Web site and store it in a database. The stock information returned includes the symbol, price, and change in a comma-separated format as follows: "XYZ",1.23,-4.5 You can, of course, use the search functions described earlier to find the commas and extract individual values with the Substring function. However, the Split function of the string class automatically separates the string into pieces. To demonstrate, you will need to set up a form: -
Add two text boxes to the form. Set the Name properties to txtInput and txtSeparator. Using labels, label these controls "Input String" and "Separator Character," respectively. -
Add a button named btnSplit. Change the caption to Split String. -
Add a list box to the form named lstOutput. -
Enter the codein Listing 6.3 in the button's Click event. Listing 6.3 STRINGEX.ZIP Parsing a String with the Split Method Dim TempArray() As String Dim CurIndex As Integer lstOutput.Items.Clear() If txtInput.Text.Length > 0 And txtSeparator.Text.Length > 0 Then TempArray = txtInput.Text.Split(txtSeparator.Text.ToCharArray) For CurIndex = 0 To TempArray.GetUpperBound(0) lstOutput.Items.Add(TempArray(CurIndex)) Next End If Run the program by pressing the F5 key. Type a sentence in the input box and a space in the separator box. Click the button and you should see results similar to those shown in Figure 6.7. Figure 6.7. With one line of code, the split function can break an string into pieces, each of which has its own array index. 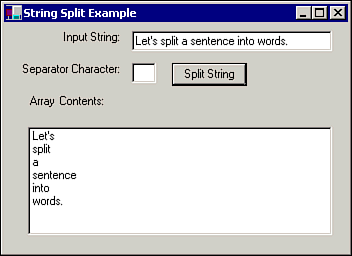 Creating a String from an Array The Join function works just the opposite of the Split function; it combines the elements in an array into a string, adding a delimiter. For the sample exercise with Join, set up another Windows Application project. Design the form as follows: -
Add a text box to the form. Set the Name property to txtArraySize and label it "Random numbers to generate:" -
Add a button beside the text box named btnJoin. Change the caption to Join Array Elements. -
Add another text box to the form. Because it may contain multiple lines of text, set its Multiline property to True and size it large enough to contain several lines of text. Set the Name property to txtOutput. -
Enter the code in Listing 6.4 in the button's Click event. Listing 6.4 STRINGEX.ZIP Creating a String from an Array Dim TempArray() As String Dim RandomInteger As New System.Random() Dim CurIndex As Integer Dim NumElements As Integer = val(txtArraySize.Text).ToInt32 'Generate random numbers, put in TempArray If NumElements > 0 Then ReDim TempArray(NumElements) For CurIndex = 0 To NumElements - 1 TempArray(CurIndex) = RandomInteger.Next(1000).tostring Next End If 'Join array elements into a comma-separated string txtOutput.text = String.Join(",", temparray) Run the program and enter a number in the text box. The For loop generates a string array of the specified size containing random numbers. The result of the Join function is shown in Figure 6.8. Figure 6.8. An example use of Join might be to combine database fields for an external program that requires a comma-separated text file. 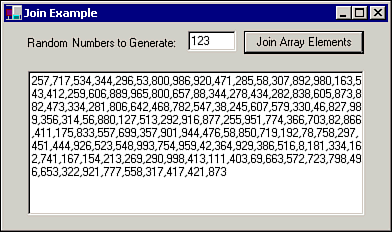 |