Customizing the development environment means making changes to the default behavior of Visual Studio to better suit an individual developer's needs. Visual Studio .NET has many new customization features available never seen before in the Visual Studio family of products. In addition to an enormous amount of user settings, Visual Studio allows the use of macros like other Microsoft products such as Excel. In this section we will describe some of the customization options you can use to make your programming experience more personalized. Changing Your Environmental Policy You can control the look and feel of Visual Studio by setting some of the many available options. To access the Options dialog box, shown in Figure 4.22, click the Tools menu and choose Options. Figure 4.22. Environment options allow developers to customize Visual Studio to suit their tastes. 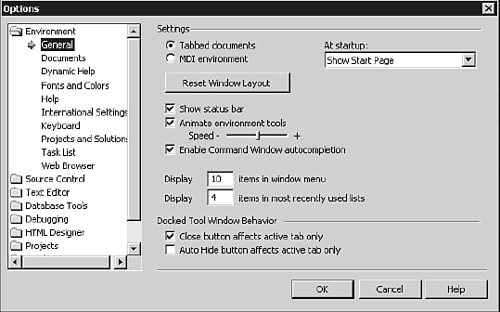 Notice that the options are organized by category using a series of folders. Because all languages in Visual Studio share the same user interface, some of the options do not apply to Visual Basic .NET. There are, in fact, so many options that discussing them all in detail would be beyond the scope of this chapter. Please feel free to experiment with the other options, but keep in mind that most exercises in this book assume the reader is using the default settings. Because the options in the Environment folder generally relate to the other topics already discussed in this chapter, the following are some highlights: General The General settings, shown in Figure 4.22, are pretty much self-explanatory. By adjusting window styles and fine-tuning animation speed, you can make the windows in your development environment behave the way you want. Documents This section controls how Visual Studio opens and displays documents. Developers will probably find the options relating to the Find box the most useful items on this screen. As with previous versions of Visual Basic, searching for text in the Code Editor (using Ctrl+F and F3) displays a message box when you transverse the entire scope of the search. By clearing the Show Message Boxes check box, you can just keep cycling through your searches continuously. The message box will not interrupt you, and the status bar will inform you when you have reached the end of your search. Dynamic Help The Dynamic Help options allow you to determine the type and order of articles displayed in the Dynamic Help window. For example, if you learn by example rather than instruction, you may want to move the Samples item to the top of the list. In this case, just single-click it and click the Move Up button. Fonts and Colors Elements in the Visual Studio environment have certain default colors text is black on a white background, comments are green, and so on. Use the options in this section to change the color scheme to your liking. Help Lets you choose to display the help windows inside or outside of the Visual Studio environment, as well as select from any of the help libraries installed on your system. International Settings Changes the language you are using. Keyboard During this chapter we have mentioned several shortcut keys; for example, F4 displays the Properties window. Using the Keyboard options here, you can change this key setting and many others. Your group of custom keyboard shortcuts can then be saved as a keyboard template. Several templates are already defined, such as those that mimic keyboard shortcuts from earlier versions of Visual Basic. (Note that this book uses the keyboard settings from either the Visual Basic or Default Settings group.) Projects and Solutions This section contains some useful options related to opening and saving projects. First, you can set your default project directory to something shorter than "My Documents," which may be useful for command-line users. You can also choose to display the Output and Task List windows automatically when a program is compiled. The Output window is frequently used for printing debug information during program execution, and the Task List as you know lists program errors. Selecting these two options means you do not have to worry about opening these windows before starting your program. The other option on this screen controls Visual Studio's behavior when saving projects. I recommend leaving it on the Save changes setting, so if your computer crashes during program execution you will not lose your changes. Task List You can add additional words, also known as tokens, that will be placed on the Task List when encountered in your program's comments. Web Browser Controls the address of the Visual Studio Home Page, the Internet Search Page, and other browsing options. Using Macros No one likes to get stuck in a rut performing the same task over and over again. Macros can help this problem by automating a group of frequently used keystrokes. For example, if you know you are always going to put a certain group of statements or comments at the beginning of every subroutine, you can create a macro to type them for you. Macros are like a tape recording of words you have typed and certain actions you performed within the development environment. Playing back the recording causes these actions to be repeated again. In this section we'll create a simple Visual Studio macro and show how to edit the code contained in the macro. To start, make sure Visual Studio is open to a new Windows application. Recording a Simple Macro Begin by placing a Button control on the form. Double-click the Button control to open the code window for the button. Place the cursor in the Click event for the button. The macro-related commands in Visual Studio are located on the Tools menu, under the Macros submenu. To begin recording a macro, click the Record Temporary Macro option now. You will see a new floating toolbar pop up the Recorder (see Figure 4.23). Figure 4.23. When recording a macro, note the cassette icon in the status bar and the appearance of the Recorder toolbar. 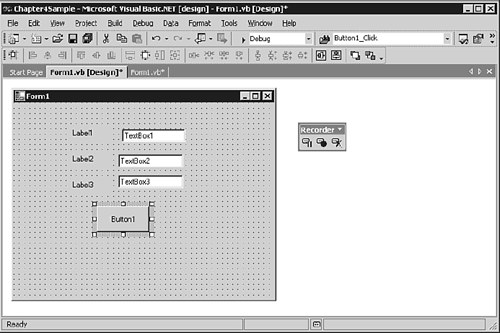 The Recorder allows you to stop and pause the recording of a macro, much like you stop or break a program during execution. To record your macro, you simply perform the activity as you would normally and the recorder will remember your keystrokes. Type the following line of code in the editor and press Enter: MessageBox.Show("Hello, World!") Next, click the Stop Recording button on the Recorder toolbar to stop recording the macro. You have now successfully recorded a macro. Executing Your Macro Visual Studio has a special window used to manage macros, the Macro Explorer, which is shown in Figure 4.24. Go ahead and display the window now by selecting the Tools menu, Macros, Macro Explorer. You should see TemporaryMacro, which is the name of the macro you just created. Figure 4.24. The Macro Explorer window can be displayed by pressing Alt+F8. 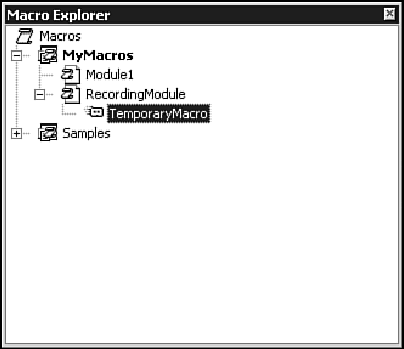 Double-click TemporaryMacro. This will run the macro you just created, and another MessageBox statement should appear in the code window. Although this macro is not very complicated, it does illustrate the basic purpose of a macro: to cut down on the amount of typing. Editing Macro Code Using the macro recorder is not the only way to create or change a macro. Macros are stored as Visual Basic code, just like your other programs, so you can easily modify the code itself. To demonstrate, right-click the TemporaryMacro in the Macro Explorer and choose Edit from the menu. A new instance of Visual Studio will appear on your screen. This screen, shown in Figure 4.25, is known as the Macros IDE. Figure 4.25. A default macro project called MyMacros is automatically loaded with Visual Studio. 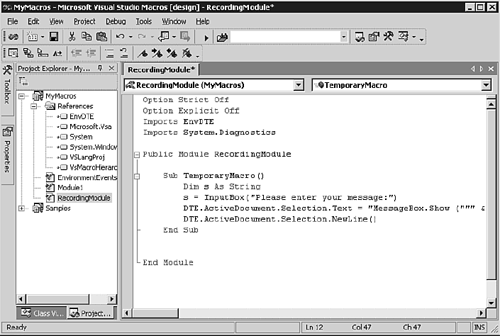 This screen should appear familiar to you. In fact, macros are really just Visual Studio projects that take advantage of the programmable aspects of Visual Studio itself. Notice the code for our macro, which should look similar to the following: Sub TemporaryMacro() DTE.ActiveDocument.Selection.Text = "MessageBox.Show(""Hello"", World!"")" DTE.ActiveDocument.Selection.NewLine() End Sub The previous code is actually very easy to follow. The DTE object, which stands for Development Tools Environment, exposes methods and properties that allow you to manipulate the Visual Studio environment with regular Visual Basic code. The first statement instructs Visual Studio to enter some text in the active document; in this case, the result is to type a MessageBox statement into our code window. The second line of code inserts a new line. Let's modify the code and see how the results affect our macro. Change the code in the macro so it looks like the following: Sub TemporaryMacro() Dim s As String s = InputBox("Please enter your message:") DTE.ActiveDocument.Selection.Text = "MessageBox.Show (""" & s & """)" DTE.ActiveDocument.Selection.NewLine() End Sub Now, close the Macros IDE and return to your Visual Studio project. Double-click the macro name (TemporaryMacro) in the Macro Explorer. An input box will appear asking you to type in a message. Enter some text and a MessageBox statement will be generated that includes the text. Tip In addition to aiding in coding tasks, the DTE object can be useful in managing projects and files. To learn more, view the code for the many sample macros included with Visual Studio .NET. Organizing Your Macros The macro we created in the previous section was called TemporaryMacro. As you might imagine, the next time you choose the Record Temporary Macro option from the menu the previous macro will be erased. To prevent this, you can rename the macro using the Macro Explorer. To complete our sample exercise, go ahead and rename your new macro. Right-click the word TemporaryMacro and choose Rename from the menu. Change the name of the macro to NewMessageBox. Now, when you create a new temporary macro, this macro will not be overwritten. As you saw in the previous section, the code for macros is stored in a project. This is a special type of project, known as a Macro Project. As you can see from Figure 4.25, the name of the default the Macro project is MyMacros. You can load or create other Macro projects in the Macro Explorer by right-clicking the word Macros in the MacroExplorer. In addition, right-clicking a code module within a macro project allows you to add and delete macros from the module. Customizing the Visual Studio Toolbars The toolbars and buttons in Visual Studio behave similarly to those found in other Microsoft products. They can be dragged and positioned to your liking on any side of the screen. This section shows how to create your own toolbar and add a button to it that will execute the sample macro we created in the previous section. To begin creating a custom toolbar, first display the Customize dialog box by clicking the View menu, Toolbars, and Customize. The Customize dialog box is shown in Figure 4.26. Figure 4.26. Visible toolbars are indicated in the Customize dialog box by a check mark next to their name. 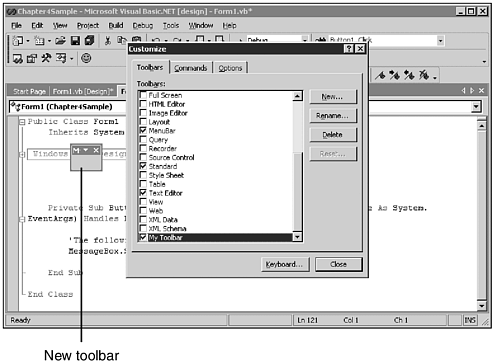 Next, press the New button and enter a name for the new toolbar, such as My Toolbar. Your toolbar will be added to the list of available toolbars and appear on the screen as a floating gray window, also pictured in Figure 4.26. In order to make your toolbar useful, you need to add a command to it. Click the Commands tab at the top of the Customize dialog box. You will see all of the available Visual Studio commands grouped by categories. Scroll through the category list until you find the Macros category, and then select it. Your macros should appear on the right side of the dialog box. If you followed the sample from the previous section, you should have a macro called NewMessageBox available. Using the mouse, drag the NewMessageBox command from the Customize dialog box to the new toolbar. Notice that the macro appears on the toolbar as text. However, it is traditional for toolbar buttons to contain images. To assign an image to your macro, right-click the macro name on the toolbar, and you should see the menu shown in Figure 4.27. Select Change Button Image, and pick one of the available images. The new image will appear on the toolbar next to the macro name. Finally, right-click again and choose Default Style to hide the macro name and show only the button image. Figure 4.27. Toolbar buttons can appear as images, text, or both. 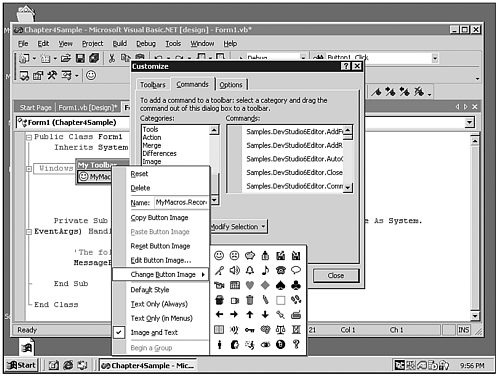 Note You can also draw custom toolbar images by clicking the Edit Button Image menu option. Note You can drag macros and other commands to existing Visual Basic toolbars, as well as custom toolbars. Tip Although the macro name is included by default, you can change the button name from the menu shown in figure 4.27. Because the button will appear as a tooltip, you may want to change it to something more descriptive. Now that you have created a new custom toolbar, close the Customize dialog box by clicking the close button. To complete our exercise, drag your new toolbar to the top of the screen so that it docks with the other toolbars. To test the toolbar, open a code window and click the button. Your macro should be executed. |