The Web Forms programming model is Microsoft's attempt to do for Web development what earlier versions of Visual Basic did for Windows development. The traditional method of programming the World Wide Web has been to write code on a Web server that generates HTML for the client browser. This approach (which we covered in the last chapter) is perfectly functional, but not very elegant. Let's examine why, by looking at the code you would need to allow a user to update his address via a Web page. In a traditional Web application, you would have to write the following code: You need to access the database and generate the initial HTML form for the end user. First, you use ADO.NET to get the user's address and store it as a variable. (ADO.NET is a set of classes that allow your code to access a database.) Next, as the page is generated and sent to the browser, your code creates the HTML form on the fly by setting up the address field with a Response.Write statement: Response.Write("<INPUT TYPE=""TEXT"" NAME=""txtAddress"" VALUE=""" & _ strAddress & """>") After the user has updated the text field, he will submit the HTML form back to the server for processing. Your code will then retrieve the address from the HTML field and update the database. You will also need to handle validating the data for errors and drawing the page again if necessary. For more on database access with ADO.NET, p.599 There is no doubt about it, the method of writing Web applications that I have just described works. There have been many ASP success stories. However, the drawback is that the code structure is often a spaghetti mixture of user interface elements and business logic. You can alleviate this problem somewhat, through the use of compiled business objects accessed from the ASP code. However, to make a Web page act as useful as a Windows form, you still have to get involved in state management, and other request and response communication issues. In short, with a traditional ASP application there is no way of avoiding a lot of messy HTML and unstructured code. Web Forms, on the other hand, provide a clean separation between the user interface and application code, as well as a familiar control-based programming model. Here's how you would create a Web Form for the user to update his address: Instead of using a traditional INPUT field to represent the address field, Web Forms allow you to use a server-side control, in this case a text box: <asp:TextBox runat="server"></asp:TextBox> If you are already familiar with HTML tags, you might notice the HTML in the previous line of code looks a little different. That is because it is not really standard HTML; instead it represents a server-side control to the ASP.NET framework. However, if you use Visual Studio .NET to create your Web form, you do not have to learn any of the new server-side control syntax. When the page loads, you can populate the field by setting the Text property of the control: txtAddress.Text = strAddress Finally, when the user submits the form, you can retrieve the updated address from the control just as easily: strNewAddress = txtAddress.Text If the previous steps sound familiar, they should. When you program using the Web Forms model, you work with events and properties of a Web page and its components just as you would with those of a Windows form. In Chapter 2, "Creating Your First Windows Application," and Chapter 3, "Creating Your First Web Application," we built a loan payment calculator using Windows Forms in the former chapter and Web Forms in the latter. In each case, we wrote code that executed in response to events. (If you have not already completed the Loan Calculator exercise, it might be a good idea to do so now, so the discussion of Web Forms and controls that follows will make more sense.) For a step-by-step example of designing a Web Form, p.49 Understanding Server Controls The Web Forms model makes extensive use of server-side controls. ASP.NET server-side controls are components that execute on the Web server and are designed to isolate the developer from the underlying HTML forms. Consider our earlier example of a text box: <asp:TextBox runat="server"></asp:TextBox> Note the <asp:> and </asp:> tags, which indicate an ASP.NET server control. It is important to realize that server controls are only understood by the server; standard HTML is still sent to the browser. Figure 18.1 shows two views of the HTML source for the Loan Calculator program. The file on top is the original .aspx file as generated by Visual Studio. The file on the bottom is what happens when the user chooses View Source in his browser. Figure 18.1. ASP Server controls do not require any special client components, because they are translated into standard HTML by the ASP.NET page processor. 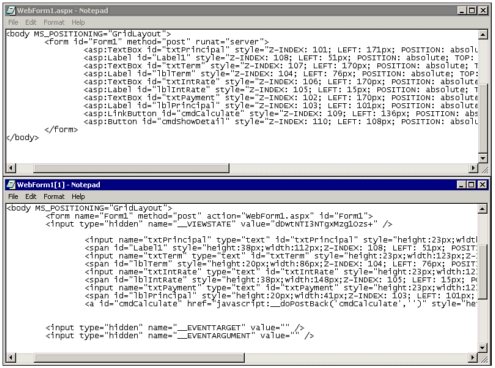 In the figure, you can see where the <asp:TextBox> tags were replaced with standard HTML <INPUT> fields. What this tells you is that the Web Forms programming model really does not do anything to change the way the Web works, it is strictly an aid to the developer. Each time a browser requests a Web page from a server, the server still has to generate an HTML page. Form Posting and Round Trips Web applications are thought of as "thin client" applications, because all the processing logic typically resides on the server. Of course, this means that any updates to the page require a round trip; that is a request followed by a response. One particular type of request from the browser is a post, which means form field data is posted to the server with the Web page request. In a traditional ASP application, the developer has to write his or her own code to maintain form state across each round trip. For example, consider a Web page that requires data entry in thirty fields. Suppose the end user makes a mistake in one of the fields, which prevents the application from processing the form. In a Windows application, whatever the user had already typed in the other twenty-nine fields will still be there, because the form is only loaded once. In a browser-based application, however, the round trip will require the server to send a new page back to the client. A well-written application should of course not just send back a blank HTML form, but also include the data the user has already entered. In other words, maintaining a form's state over round trips means the user will only have to retype the incorrect value instead of all 30. When you program using the Web Forms model, this problem goes away, thanks to something called view state. If you look at Figure 18.1 again, you will see that in addition to translating the ASP server controls into their equivalent HTML elements, the ASP.NET page processor also added some hidden form fields and other elements for its internal use. Notice the VIEWSTATE hidden field, which appears to be an unrecognizable string of characters. This field is generated automatically and used to maintain the values of your form fields across multiple round trips. Thanks to the view state feature, you do not have to code this yourself. Once the end user keys a value into a text box, the value will remain in the text box, regardless of how many round-trips the page makes, until your code changes it or he moves to another Web page. Note Maintaining field data over several round trips for a single Web form (view state) is a different issue from maintaining information across several Web pages (session state). For more information, see the upcoming section "Distinguishing Between View State and Session State." |