Recipe 3.3. Deploying a Java Servlet That Uses AspectJ Problem You want to deploy a servlet that has been developed using AspectJ into Apache Tomcat. Solution Compile your Java Servlet from the command line using the ajc command or inside an Eclipse AspectJ project. Under the webapps directory inside Apache Tomcat, set up a new web application directory and WEB-INF subdirectory. Make the appropriate amendments to the server.xml file in the Tomcat configuration to enable your web application. Copy the compiled Java Servlet .class files and corresponding aspect .class files into the webapps/%YOUR_APPLICATION_DIRECTORY%/WEB-INF/classes. Copy the aspectjrt.jar file into the webapps/%YOUR_APPLICATION_DIRECTORY%/WEB-INF/lib so the aspect-oriented features of your software can find the support components they need. Amend your web application's webapps/%YOUR_APPLICATION_DIRECTORY/WEB-INF/web.xml file to support access to the new Java Servlet. Finally, restart Tomcat to activate your web application. Discussion The following steps show how to create, compile, and deploy a simple Java Servlet that uses AspectJ: Create a Java Servlet and corresponding aspect similar to the ones shown in Examples Example 3-2 and Example 3-3. Example 3-2. A simple HelloWorld Java Servlet package com.oreilly.aspectjcookbook; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.ServletOutputStream; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class AOHelloWorldServlet extends HttpServlet { public void doGet (HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { ServletOutputStream out = response.getOutputStream( ); out.println("<h1>Hello World from an aspect-oriented Servlet!</h1>"); } public String getServletInfo( ) { return "Create a page that says <i>Hello World</i> and send it back"; } } Example 3-3. An aspect that advises the doGet(..) method on the AOHelloWorldServlet class package com.oreilly.aspectjcookbook; import java.io.IOException; import javax.servlet.ServletOutputStream; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public aspect AddHTMLHeaderAndFooter { public pointcut captureHttpRequest(HttpServletRequest request, HttpServletResponse response) : execution(public void AOHelloWorldServlet.doGet(HttpServletRequest, HttpServletResponse)) && args(request, response); before(HttpServletRequest request, HttpServletResponse response) throws IOException : captureHttpRequest(request, response) { response.setContentType("text/html"); ServletOutputStream out = response.getOutputStream( ); out.println("<html>"); out.println("<head><title>Adding a title using AspectJ!</title></head>"); out.println("<body>"); } after(HttpServletRequest request, HttpServletResponse response) throws IOException : captureHttpRequest(request, response) { ServletOutputStream out = response.getOutputStream( ); out.println("</body>"); out.println("</html>"); } } Compile your Java Servlet and aspect as normal using either the ajc command-line tool or as part of an AspectJ project in Eclipse. Create a new web application directory in Tomcat by creating a subdirectory of %TOMCAT_INSTALL_DIRECTORY%/webapps called mywebapplication. Create a subdirectory of mywebapplication called WEB-INF that contains a classes and lib directory. Create a new file inside mywebapplication/WEB-INF called web.xml that has the following contents: <?xml version="1.0" encoding="ISO-8859-1"?> <!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd"> <web-app> <display-name>MyApplication</display-name> <description> A simple web application that demonstrates the deployment of Java Servlets that have been built using AspectJ. </description> <servlet> <servlet-name>AOHelloWorld</servlet-name> <servlet-class>com.oreilly.aspectjcookbook.AOHelloWorldServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>AOHelloWorld</servlet-name> <url-pattern>/AOHelloWorld</url-pattern> </servlet-mapping> </web-app> Copy the .class files generated from your compilation of the Java Servlet and aspect to the mywebapplication/WEB-INF/classes directory remembering to preserve the package directory structure. Copy the aspectjrt.jar from %ASPECTJ_INSTALL_DIRECTORY%/lib to myweb-application/WEB-INF/lib. For convenience, create a new file inside mywebapplication called index.html that has the following contents: <html> <title>Homepage for MyApplication</title> <body> <h1> Testing hompage for aspect-oriented Java Servlets</h1> <p> The purpose of this page is to simply provide a set of links with which to conveniently access your aspect-oriented Java Servlets. </p> <p> <ul> <li> <a href="AOHelloWorld"> The simple HelloWorld aspect-oriented Java Servlet </a> </li> </ul> </p> </body> </html> This is an optional step that provides an HTML page that can be used to access your Java Servlet conveniently from the browser without having to manually enter its specific URL. The directory structure for your deployed web application should look like that shown in Figure 3-5. Figure 3-5. The deployed web application directory and file structure 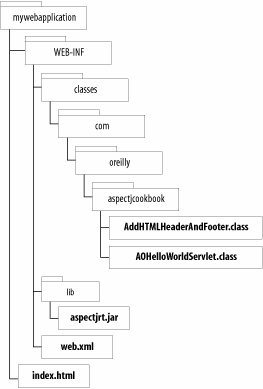
Edit the %TOMCAT_INSTALL_DIRECTORY%/conf/server.xml and add the following lines to enable your web application inside Tomcat: <Context path="/mywebapplication" docBase="mywebapplication" debug="0" reloadable="true" crossContext="true"> </Context> Finally, restart Tomcat, and you should be able to access your web application and its Java Servlets using your browser by entering a URL similar to :/mywebapplication/index.html">http://<HOSTNAME>:<TOMCAT_PORT>/mywebapplication/index.html, entering the hostname and Tomcat port number for your installation of Tomcat. Figure 3-6 shows the homepage for your web application. Figure 3-6. Your web applications homepage 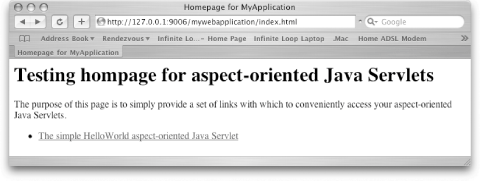
By clicking on the "The simple HelloWorld aspect-oriented Java Servlet" link on your web applications homepage, your browser should show the output of your Java Servlet, as shown in Figure 3-7. Figure 3-7. The output from your aspect-oriented Java Servlet 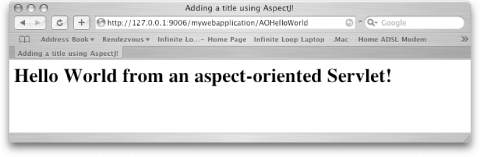
See Also Compiling using the ajc command-line tool is explained in Recipe 2.2; creating and compiling an AspectJ project in Eclipse is described in Recipe 2.7; the execution(Signature) pointcut is explained in Recipe 4.4; the args([TypePatterns | Identifiers]) pointcut is explained in Recipe 11.3; the before( ) form of advice is discussed in Recipe 13.3; the after( ) form of advice is shown in Recipe 13.5; Java Servlet & JSP Cookbook by Bruce W. Perry (O'Reilly) contains examples of how to configure and deploy your Java Servlets in Tomcat and other containers; Tomcat: The Definitive Guide by Jason Brittain and Ian Darwin (O'Reilly). |