The highly distributed nature of the integration capabilities of the ESB is largely due to traits of the ESB service container. A service container is the physical manifestation of the abstract endpoint, and provides the implementation of the service interface. A service container is a remote process that can host software components. In that respect, it has some similarities to an application server container, but with the specific goal of hosting integration services. A service container is simple and lightweight, but it can have many discrete functions. As illustrated in Figure 6-10, service containers take on different roles as they are deployed across an ESB. Figure 6-10. ESB service containers are specialized for integration services such as transformation, routing, and application adapters 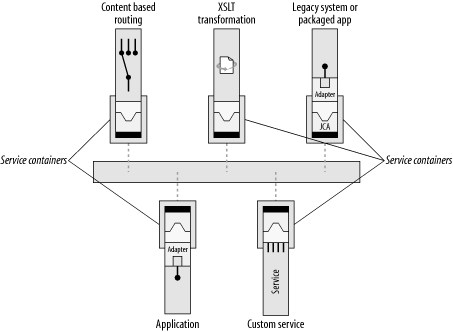 Unlike its distant cousins, the J2EE application server container and the EAI broker, the ESB service container allows the selective deployment of integration broker functionality exactly when and where you need it, and nothing more than what you need. In its simplest state, a service container is an operating system process that can be managed by the ESB's invocation and management framework. A service container can host a single service, or can combine multiple services in a single container environment, as illustrated in Figure 6-11. Figure 6-11. The ESB service container allows selective deployment of a single service, or can be combined with other services 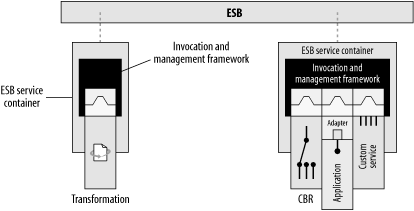 An ESB service is also scalable in a fashion that is independent of all other ESB services. A service container may manage multiple instances of a service within a container. Several containers may also be distributed across multiple machines for the purposes of scaling up to handle increased message volume (Figure 6-12). Figure 6-12. Services may be scaled within a container, and several containers may be scaled across multiple machines 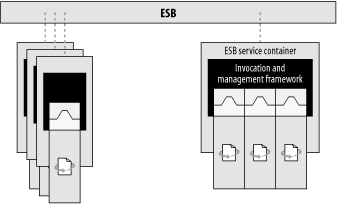 Is XML Too Slow? One of the most common observations regarding XML is its potential to consume computing resources due to its verbosity. An XML business document that represents an EDI document can range from 110 KB to more than 100 MB in size (often five times larger than the original EDI message), and can consume a great deal of CPU and memory while being parsed and translated. Deploying multiple instances of a particular XSLT transformation service across many machines can provide a very powerful and flexible scaling capability. If the implementation of the service containers is portable across multiple platforms, you can also take advantage of existing hardware assets. Imagine having the flexibility to commandeer a set of Linux, AIX, and Win2K servers for the purpose of spreading out the load of a particular XML transformation. In addition, because the service containers are relatively lightweight, you can take advantage of less powerful machines that may be underutilized within your organization. |
6.6.1 The Management Interface of the Service Container An ESB service container should handle the inflow and outflow of management data such as configuration, auditing, and fault handling. Management interfaces should be implemented using the Java Management eXtensions (JMX) if the ESB implementation supports Java. A more detailed discussion on the use of JMX in an ESB can be found in Chapter 10. As illustrated in Figure 6-13, an ESB service container supports the retrieval of configuration data from a directory service, and can also have a local cache of configuration data. This means that even if other parts of the ESB, including the directory service, become temporarily unavailable, the service container can continue to operate with its current set of configuration data. Figure 6-13. Service containers support inputs and outputs for management data such as configuration, auditing, and fault handling 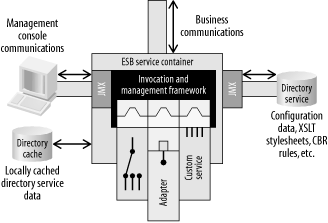 Management input data can originate from a remote management console issuing commands such as start, stop, shut down, and refresh the cache. Management output data can consist of event tracking, such as notification that a message has successfully exited the service or that a failure has occurred. These inputs and outputs can be managed by a JMX management console or redirected to some other management tool using standard protocols such as the Simple Network Management Protocol (SNMP). 6.6.2 The ESB Service Interface The ESB container provides the message flow in and out of a service. It also handles a number of facilities, such as service lifecycle and itinerary management, that we will explore in a later section. As illustrated in Figure 6-14, the container manages an entry endpoint and an exit endpoint, which are used by the container to dispatch a message to and from the service. Figure 6-14. Message dispatch to a service uses the service's configured entry and exit endpoints 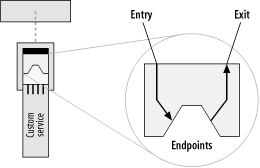 XML messages are received by the service from a configurable entry endpoint. Upon completion of its task, the service implementation simply places its output message in the exit endpoint to be carried to its next destination using the "request/reply" or "reply-forward" pattern discussed in Chapter 5. The output message may be the same message that it received. The service may modify the message before sending it to the exit endpoint. Or, the service may create a completely new message to serve as a "response" to the incoming message and send the new message in the exit endpoint. The following code example shows what a service implementation looks like: public void service(ESBServiceContext ctx) throws ESBServiceException { // Get any runtime process parameters. Parameters params = ctx.getParameters( ); // Get the message. ESBEnvelope env = null; env = ctx.getNextIncoming( ); if (env != null) { ESBMessage msg = env.getMessage( ); ... // Operate on the message } // Put the message to the Exit Endpoint ctx.addOutgoing(env); } What is placed in the exit endpoint depends on the context of the situation and the message being processed. In the case of a CBR service, the message content will be unchanged, with new forwarding addresses set in the message header. In more sophisticated cases, one input message can transform into many outputs, each with its own routing information. For example, a custom service can receive a purchase order document, split it up into multiple output messages, and send out the purchase order and its individual line items as separate messages to an inventory or order fulfillment service. The service implementation in this case does not have to be written using traditional coding practices; it can be implemented as a specialized transformation service that applies an XSLT stylesheet to the purchase order document to produce the multiple outputs (if the ESB has an XSLT extension to support multiple outputs). 6.6.3 Auditing, Logging, and Error Handling Auditing and logging play an important business function within an integration strategy. Part of the reason you want to integrate across a common backbone is to gain real-time access to the business data that is flowing between departments in an organization. A reliable communications backbone will ensure that the data gets to its intended destinations. An auditing framework will allow you to track the data at a business level. As part of its management capabilities, an ESB container provides an auditing and logging facility. This facility can have multiple sources for tracking data. System-level information about the health of the service itself and the flow of messages can be tracked and monitored. Application-level auditing, logging, and fault handling are accomplished through additional endpoints that are available to each service. As illustrated in Figure 6-15, the service implementation has three additional endpoints at its disposal: a tracking endpoint, a fault endpoint, and a rejected-message endpoint. A service can be created such that a message can be placed in the tracking endpoint in addition to its normal exit destination. A rejected-message endpoint can be used for system-level errors, such as a malformed XML document, or any case in which the service itself throws an exception. The fault endpoint can be used for any application-level errors, or faults, that can occur. Figure 6-15. Additional tracking and fault handling endpoints are handled by the service container 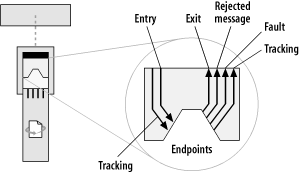 There is an underlying philosophy behind the way tracking, system errors, and application faults are handled. In addition to a normal exit endpoint to handle the outgoing flow of a message, additional destinations are available to the service for auditing the message and for reporting errors. The service implementation has these endpoints available for its own use. From the service implementation's point of view, it simply places data into the tracking endpoint or fault endpoint, and the surrounding ESB framework takes care of managing the auditing, logging, and error reporting. A tracking endpoint can be configured to point to anything a topic or queue destination, or even a call to an external web service. This approach provides a separation between the implementation of the service and the details surrounding the fault handling. The implementer of a service need only be concerned that it has a place to put such information, whether it is information concerning the successful processing of good data, or the reporting of errors and bad data. Tracking can be handled at both the individual service level and the business process level. A business process may make use of different implementations of individual services over time. The tracking of a fault occurrence or the auditing of an individual message can be tied to the context of the greater business process that is utilizing the service at the time. In Chapter 7, we will explore more about how auditing facilities are used. We will also see that the use of these endpoints is one way of doing auditing and logging with an ESB. Other approaches include placing a specialized XML persistence service in the path of a message in a process flow, which can be much less intrusive to each individual service implementation. 6.6.4 ESB Service Container Facilities The container is an intrinsic part of the ESB distributed processing framework. In some sense, the container is the ESB more so than the underlying middleware that connects the containers together. The container provides the care and feeding of the service. As illustrated in Figure 6-16, the ESB container provides a variety of facilities that a service implementation may have at its disposal. Facilities such as location, routing, service invocation, and management are taken care of by the container and its surrounding framework, so the implementation of a service does not have to be concerned with those things. The container also provides facilities for lifecycle management such as startup, shutdown, and resource cleanup. Thread pooling allows multiple instances of a service to be attached to multiple listeners within a single container. Connection management facilities provide the specifics of the binding to the underlying MOM, plus additional fault tolerance and retry logic. Figure 6-16. The ESB container provides many facilities for the service implementation 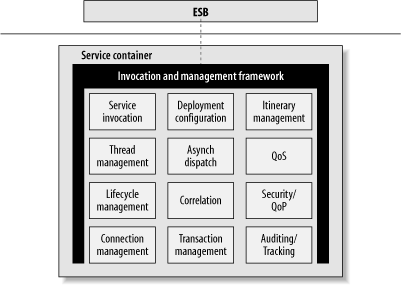 6.6.4.1 QoP and QoS Quality of Protection (QoP) and security, Quality of Service (QoS), and transaction services are also provided to the service via the ESB container. Some of these capabilities may be delegated by the service container to the underlying MOM that it is bound to. QoS options, such as exactly-once delivery of messages, are best delegated to a messaging infrastructure that is built for that. If the exactly-once delivery option is dependent on an implementation of a reliable SOAP specification such as WS-Reliability or WS-ReliableMessaging, it would likely still be delegated to a SOAP processor that has MOM qualities such as message persistence, store-and-forward, and duplicate detection. 6.6.4.2 Administration of ESB facilities All of these facilities of the ESB container are intrinsically tied to the administrative functions of the ESB, which means that even if you are writing your own custom service, these facilities are assumed to be there and don't have to be written into the service itself. Generally speaking, this type of "plumbing" is one of the reasons adopting a middleware infrastructure is so appealing. The ESB brings it to the next level by fully delegating this responsibility to the underlying "plumbing." In most cases, the container facilities don't even need to be exposed to the implementation of the service itself. The container facilities by and large represent an agreement between the container and the underlying middleware. Each facility has its own configuration nuances that can be dealt with using an administrative tool that is part of the ESB offering. For example, a QoS setting for exactly-once delivery would be set as an administrative property of a service, not something a coder would do in the API. Of course, the individual capabilities within each of these facilities are likely to vary depending on the implementation from different vendors. The method of administration of ESB facilities can range from a full-blown GUI to a command-line interface to an administrative API, depending on the implementation. Don't assume that one administrative method is "better" than another when considering implementations; each has its purpose. A command-line interface can be a good approach for those accustomed to automating processes by building things into scripts. An API is useful when trying to build an ESB into a larger infrastructure that may already have its own set of user interface administrative tools. 6.6.5 Standardizing ESB Container Connectivity Within the Java Community Process (JCP), the Java Business Integration (JBI) effort is underway to standardize the means by which components plug into an integration infrastructure. For an ESB, this will provide conformity across implementations. More details on JBI can be found in Chapter 10. |