6.7. Unleash the Console .NET 1.0 introduced the Console class to give programmers a convenient way to build simple command-line applications. The first version of the Console was fairly rudimentary, with little more than basic methods like Write( ), WriteLine( ), Read( ), and ReadLine( ). In .NET 2.0, new features have been added, allowing you to clear the window, change foreground and background colors, alter the size of the window, and handle special keys. Note: At last, a Console class with keyboard-handling and screen-writing features. 6.7.1. How do I do that? The best way to learn the new features is to see them in action. Example 6-7 shows a simple application, ConsoleTest, which lets the user move a happy face character around a console window, leaving a trail in its wake. The application intercepts each key press, checks if an arrow key was pressed, and ensures that the user doesn't move outside of the bounds of the window. Warning: In order for the advanced console features to work, you must disable the Quick Console window. The Quick Console is a console window that appears in the design environment, and it's too lightweight to support features like reading keys, setting colors, and copying characters. To disable it, select Tools Options, make sure the "Show all settings checkbox" is checked, and select the Debugging General tab. Then, turn off the "Redirect all console output to the Quick Console window." Example 6-7. Advanced keyboard handling with the console Module ConsoleTest Private NewX, NewY, X, Y As Integer Private BadGuyX, BadGuyY As Integer Public Sub Main( ) ' Create a 50 column x 20 line window. Console.SetWindowSize(50, 20) Console.SetBufferSize(50, 20) ' Set up the window. Console.Title = "Move The Happy Face" Console.CursorVisible = False Console.BackgroundColor = ConsoleColor.DarkBlue Console.Clear( ) ' Display the happy face icon. Console.ForegroundColor = ConsoleColor.Yellow Console.SetCursorPosition(X, Y) Console.Write(" ") ' Read key presses. Dim KeyPress As ConsoleKey Do KeyPress = Console.ReadKey( ).Key ' If it's an arrow key, set the requested position. Select Case KeyPress Case ConsoleKey.LeftArrow NewX -= 1 Case ConsoleKey.RightArrow NewX += 1 Case ConsoleKey.UpArrow NewY -= 1 Case ConsoleKey.DownArrow NewY += 1 End Select MoveToPosition( ) Loop While KeyPress <> ConsoleKey.Escape ' Return to normal. Console.ResetColor( ) Console.Clear( ) End Sub Private Sub MoveToPosition( ) ' Check for an attempt to move off the screen. If NewX = Console.WindowWidth Or NewX < 0 Or _ NewY = Console.WindowHeight Or NewY < 0 Then ' Reset the position. NewY = Y NewX = X Console.Beep( ) Else ' Repaint the happy face in the new position. Console.MoveBufferArea(X, Y, 1, 1, NewX, NewY) ' Draw the trail. Console.SetCursorPosition(X, Y) Console.Write("*") ' Update the position. X = NewX Y = NewY Console.SetCursorPosition(0, 0) End If End Sub End Module To try this out, run the application and use the arrow keys to move about. Figure 6-2 shows the output of a typical ConsoleTest session. Figure 6-2. A fancy console application 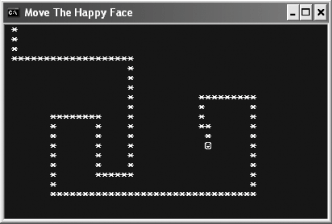 Some of the new Console methods used in ConsoleTest include the following:
- Clear( )
-
Erases everything in the console window and positions the cursor in the top-left corner.
- SetCursorPosition( )
-
Moves the cursor to the designated x- and y-coordinates (measured from the top-left corner). Once you've moved to a new position, you can use Console.Write( ) to display some characters there.
- SetWindowSize( ) and SetBufferSize( )
-
Allow you to change the size of the window (the visible area of the console) and the buffer (the scrollable area of the console, which is equal to or greater than the window size).
- ResetColor( )
-
Resets the foreground and background colors to their defaults.
- Beep( )
-
Plays a simple beep, which is often used to indicate invalid input.
- ReadKey( )
-
Reads just a single key press and returns it as a ConsoleKeyInfo object. You can use this object to easily tell what key was pressed (including extended key presses like the arrow keys) and what other keys were held down at the time (like Alt, Ctrl, or Shift).
- MoveBufferArea( )
-
Copies a portion of the console window to a new position, and erases the original data. This method offers a high-performance way to move content around the console. The new Console properties include:
- Title
-
Sets the window caption.
- ForegroundColor
-
Sets the text color that will be used the next time you use Console.Write( ) or Console.WriteLine( ).
- BackgroundColor
-
Sets the background color that will be used the next time you use Console.Write( ) or Console.WriteLine( ). To apply this background color to the whole window at once, call Console.Clear() after you set the background color.
- CursorVisible
-
Hides the blinking cursor when set to False.
- WindowHeight and WindowWidth
-
Returns or sets the dimensions of the console window.
- CursorLeft and CursorTop
-
Returns or moves the current cursor position. 6.7.2. What about... ...reading a character from a specified position of the window? Sadly, the new Console class provides no way to do this. That means that if you wanted to extend the happy face example so that the user must navigate through a maze of other characters, you would need to store the position of every character in memory (which could get tedious) in order to check the requested position after each key press, and prevent the user from moving into a space occupied by another character. 6.7.3. Where can I learn more? To learn more about the new Console class and its new properties and methods, look for the Console and ConsoleKeyInfo classes in the MSDN help library reference. |