Nest the following if/else statement in the "up" leg of the if/else if statement you just entered in Step 6:
if (display_mc.list_mc._y - speed + display_mc.list_mc._height > (startingY + bottom)) { display_mc.list_mc._y -= speed; } else { display_mc.list_mc._y = (startingY + bottom) - display_mc.list_mc._height; }
Because this statement is nested within the "up" leg of the previous statement, it's evaluated if the value of direction is up.
The first part of the expression in the statement is used to determine what the bottom position of the list_mc instance would be if it were to move 10 pixels upward. The expression does this by looking at the list_mc instance's current y position and then subtracting the value of speed and adding the height of the instance. That bottom position of the instance is then compared against one of the scrolling boundaries, as established by adding the value of bottom to the value of startingY. If moving the list_mc instance up doesn't cause the bottom of the instance to exceed the boundary, the first action in the statement is executed and the list is moved up. If moving the instance up would make it exceed the boundary, however, the else part of the statement would be executed, simply snapping its vertical position to the maximum allowable.
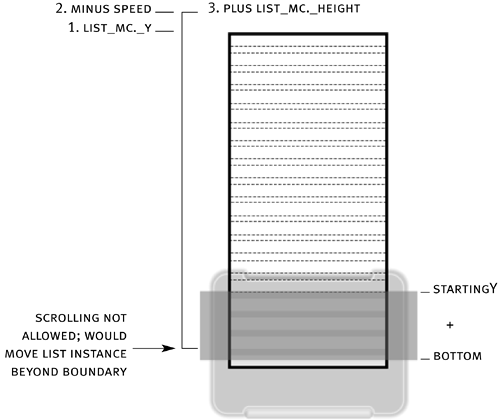
If you're confused, don't worry: Let's look at an example to see how this technique works. For this demonstration, we'll assume the following values:
var speed:Number = 10 var startingY:Number = 100 var bottom:Number = 120 display_mc.list_mc._height = 400 //vertical height of the list instance display_mc.list_mc._y = -165 //current vertical position of the list instance
Using these values, the expression in the if statement is evaluated as follows:
if (-165 - 10 + 400 > (100 + 120))
which further evaluates to:
if (225 > 220)
In this case, 225 is greater than 220, so the following action is executed:
display_mc.list_mc._y -= speed;
This moves the list_mc instance up 10 pixels, and its y position now equals 175. If the statement were evaluated again, it would look like this:
if (-175 - 10 + 400 > (100 + 120))
which further evaluates to:
if (215 > 220)
In this case, 215 is less than 220, so the following action (the else part of the statement) is executed:
display_mc.list_mc._y = (startingY + bottom) - display_mc.list_mc._height;
Here, the list_mc instance's y position is set based on the value of the expression to the right of the equals sign. Using the values established for this example, this expression would be evaluated as follows:
(startingY + bottom) - display_mc.list_mc._height
which further evaluates to:
(100 + 120) - 400
which further evaluates to:
220 - 400
which further evaluates to:
-180
This means that the list_mc instance would be snapped into a vertical position of 180, placing the bottom of the instance at the edge of the upper scrolling boundary. This statement is set up so that the bottom of the list_mc instance never scrolls beyond this point.
NOTE
This can be a tricky concept: You may want to review this information several times.
Next, let's set up the function to handle downward scrolling.