Forms Web applications run on form submissions, and JSF applications are no exception. Table 4-7 lists all h:form attributes. Table 4-7. Attributes for h:form Attributes | Description | prependId (JSF 1.2) | true (default) if the ID of this form is prepended to the IDs of its components; false to suppress prepending the form ID (useful if the ID is used in JavaScript code) | binding, id, rendered, styleClass | Basic attributes | accept, acceptcharset, dir, enctype, lang, style, target, title | HTML 4.0 attributes | onclick, ondblclick, onfocus, onkeydown, onkeypress, onkeyup, onmousedown, onmousemove, onmouseout, onmouseover, onmouseup, onreset, onsubmit | DHTML events | Although the HTML form tag has method and action attributes, h:form does not. Because you can save state in the client an option that is implemented as a hidden field posting forms with the GET method is disallowed. The contents of that hidden field can be quite large and may overrun the buffer for request parameters, so all JSF form submissions are implemented with the POST method. There is no need for an anchor attribute since JSF form submissions always post to the current page. (Navigation to a new page happens after the form data have been posted.) The h:form tag generates an HTML form element. For example, if, in a JSF page named /index.jsp, you use an h:form tag with no attributes, the Form renderer generates HTML like this: <form method="post" action="/forms/faces/index.jsp" enctype="application/x-www-form-urlencoded"> h:form comes with a full complement of DHTML event attributes. You can also specify the style or styleClass attributes for h:form. Those styles will then be applied to all output elements contained in the form. Finally, the id attribute is passed through to the HTML form element. If you do not specify the id attribute explicitly, a value is generated by the JSF implementation, as is the case for all generated HTML elements. The id attribute is often explicitly specified for forms so that it can be referenced from style sheets or scripts. Form Elements and JavaScript JavaServer Faces is all about server-side components, but it is also designed to work with scripting languages, such as JavaScript. For example, the application shown in Figure 4-1 uses JavaScript to confirm that a password field matches a password confirm field. If the fields do not match, a JavaScript dialog is displayed. If they do match, the form is submitted. We use the id attribute to assign names to the relevant HTML elements so that we can access them with JavaScript: <h:form > ... <h:inputText .../> <h:inputText .../> ... <h:commandButton type="button" onclick="checkPassword(this.form)"/> ... </h:form> When the user clicks the button, a JavaScript function checkPassword is invoked, as follows: function checkPassword(form) { var password = form["registerForm:password"].value; var passwordConfirm = form["registerForm:passwordConfirm"].value; if (password == passwordConfirm) form.submit(); else alert("Password and password confirm fields don't match"); } Notice the syntax used to access form elements. You might think you could access the form elements with a simpler syntax, like this: documents.forms.registerForm.password But that will not work. Now we look at the HTML produced by the preceding code to find out why: <form method="post" action="/javascript/faces/index.jsp" enctype="application/x-www-form-urlencoded"> ... <input type="text" name="registerForm:password"/> ... <input type="button" name="registerForm:_id5" value="Submit Form" onclick="checkPassword(this.form)"/> ... </form> All form controls generated by JSF have names that conform to formName:componentName where formName represents the name of the control's form and componentName represents the control's name. If you do not specify id attributes, the JSF framework creates identifiers for you, as you can see from the button in the preceding HTML fragment. Therefore, to access the password field in the preceding example, you must do this instead: documents.forms.registerForm["registerForm:password"].value The directory structure for the application shown in Figure 4-1 is shown in Figure 4-2. The JSF page is listed in Listing 4-1 and the English resource bundle is listed in Listing 4-2. 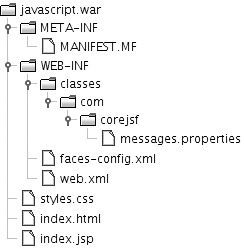 Listing 4-1. javascript/web/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <title> 7. <h:outputText value="#{msgs.windowTitle}"/> 8. </title> 9. </head> 10. <body> 11. <h:form > 12. <table> 13. <tr> 14. <td> 15. <h:outputText value="#{msgs.namePrompt}"/> 16. </td> 17. <td> 18. <h:inputText/> 19. </td> 20. </tr> 21. <tr> 22. <td> 23. <h:outputText value="#{msgs.passwordPrompt}"/> 24. </td> 25. <td> 26. <h:inputSecret /> 27. </td> 28. </tr> 29. <tr> 30. <td> 31. <h:outputText value="#{msgs.confirmPasswordPrompt}"/> 32. </td> 33. <td> 34. <h:inputSecret /> 35. </td> 36. </tr> 37. </table> 38. <h:commandButton type="button" value="Submit Form" 39. onclick="checkPassword(this.form)"/> 40. </h:form> 41. </body> 42. <script type="text/javascript"> 43. <!-- 44. function checkPassword(form) { 45. var password = form["registerForm:password"].value; 46. var passwordConfirm = form["registerForm:passwordConfirm"].value; 47. 48. if(password == passwordConfirm) 49. form.submit(); 50. else 51. alert("Password and password confirm fields don't match"); 52. } 53. --> 54. </script> 55. </f:view> 56. </html>
| Listing 4-2. javascript/src/java/com/corejsf/messages.properties 1. windowTitle=Accessing Form Elements with JavaScript 2. namePrompt=Name: 3. passwordPrompt=Password: 4. confirmPasswordPrompt=Confirm Password:
| |