Bean Scopes For the convenience of the web application programmer, a servlet container provides separate scopes, each of which manages a table of name/value bindings. These scopes typically hold beans and other objects that need to be available in different components of a web application. Session Scope Recall that the HTTP protocol is stateless. The browser sends a request to the server, the server returns a response, and then neither the browser nor the server has any obligation to keep any memory of the transaction. This simple arrangement works well for retrieving basic information, but it is unsatisfactory for server-side applications. For example, in a shopping application, you want the server to remember the contents of the shopping cart. For that reason, servlet containers augment the HTTP protocol to keep track of a session that is, repeated connections by the same client. There are various methods for session tracking. The simplest method uses cookies: name/value pairs that a server sends to a client, hoping to have them returned in subsequent requests (see Figure 2-6). 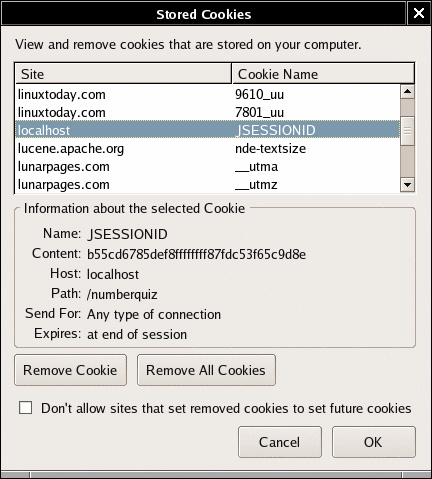 As long as the client does not deactivate cookies, the server receives a session identifier with each subsequent request. Application servers use fallback strategies, such as URL rewriting, for dealing with those clients that do not return cookies. URL rewriting adds a session identifier to a URL, which looks somewhat like this: http://corejsf.com/login/index.jsp;jsessionid=b55cd6...d8e Note | To see this behavior, tell your browser to reject cookies from the localhost, then restart the web application and submit a page. The next page will have a jsessionid attribute. | Session tracking with cookies is completely transparent to the web developer, and the standard JSF tags automatically perform URL rewriting if a client does not use cookies. The session scope persists from the time that a session is established until session termination. A session terminates if the web application invokes the invalidate method on the HttpSession object, or if it times out. Web applications typically place most of their beans into session scope. For example, a UserBean can contain information about users that is accessible throughout the entire session. A ShoppingCartBean can be filled up gradually during the requests that make up a session. Application Scope The application scope persists for the entire duration of the web application. That scope is shared among all requests and all sessions. You place managed beans into the application scope if a single bean should be shared among all instances of a web application. The bean is constructed when it is first requested by any instance of the application, and it stays alive until the web application is removed from the application server. Request Scope The request scope is short-lived. It starts when an HTTP request is submitted and ends when the response is sent back to the client. If you place a managed bean into request scope, a new instance is created with each request. Not only is this is potentially expensive, it is also not appropriate if you want your data to persist beyond a request. You would place an object into request scope only if you wanted to forward it to another processing phase inside the current request. For example, the f:loadBundle tag places the bundle variable in request scope. The variable is needed only during the Render Response phase in the same request. Caution | Only request scope beans are single-threaded and, therefore, inherently threadsafe. Perhaps surprisingly, session beans are not single-threaded. For example, a user can simultaneously submit responses from multiple browser windows. Each response is processed by a separate request thread. If you need thread safety in your session beans, you should provide locking mechanisms. | Life Cycle Annotations Starting with JSF 1.2, you can specify managed bean methods that are automatically called just after the bean has been constructed and just before the bean goes out of scope. This is particularly convenient for beans that establish connections to external resources such as databases. Annotate the methods with @PostConstruct or @PreDestroy, like this: public class MyBean { @PostConstruct public void initialize() { // initialization code } @PreDestroy public void shutdown() { // shutdown code } // other bean methods } These methods will be automatically called, provided the web application is deployed in a container that supports the annotations of JSR (Java Specification Request) 250 (see http://www.jcp.org/en/jsr/detail?id=250). In particular, Java EE 5-compliant application servers such as GlassFish support these annotations. It is expected that standalone containers such as Tomcat will also provide support in the future. |