You embed the custom tag into a JSP using syntax that is similar to other actions. <%@ taglib uri="xyz-taglib.tld" prefix="xyz" %> This associates the tags in the taglib with the name (prefix) xyz. If you had a tag called myTag defined in the .tld file, you would add it to your JSP using the following syntax: ... <body> <xyz:myTag /> </body> ... The meaning of the tag is defined in the Tag Library Definition file (.tld). The .tld file will contain a number of lines of boilerplate code followed by the definition of one or more tags using the following syntax: ... <tag> <name>myTag</name> <tagclass>com.samspublishing.jpp.ch25.MyTag</tagclass> <info>This tag does xyz</info> <bodycontent>EMPTY</bodycontent> </tag> ... The tagclass is the name of the class in the classpath of the server. It will be a normal Java class that extends one of the helper classes mentioned previously. It provides an implementation of the doStartTag() method, which is required by the Tag interface. The basic structure of this class file is shown here: package com.samspublishing.jpp.ch25; import javax.servlet.jsp.tagext.*; . . . public class MyTag extends TagSupport { public int doStartTag() { create some interesting output } return(SKIP_BODY) } The combination of these three parts is shown in Figure 24.1. Figure 24.1. The three components of the custom tag are the JSP file, the Tag Library Descriptor (.tld) file, and the class file. 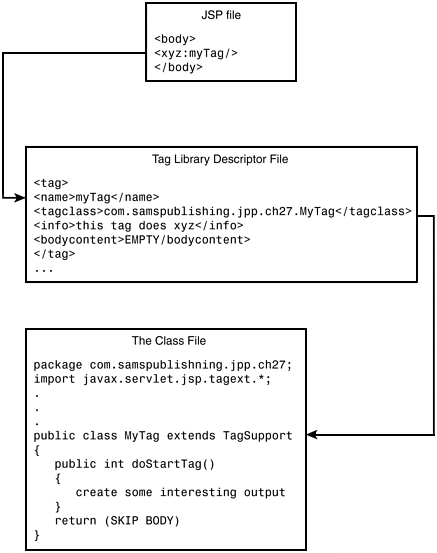 The JSP file uses the name of the tag. The JSP engine looks up the tagclass at runtime and runs the doStartTag() method in this class that provides the output that will be inserted in the JSP. A simple example will illustrate how this works. We need to create a tag that can be included in a JSP. We'll call this tag stringTag. Before we can start using stringTag, we have to create a tag library, or taglib, to define the behavior of the tag. Listing 24.1 shows the contents of a taglib. Listing 24.1 The jpptaglib.tld File <?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE taglib PUBLIC "-//Sun Microsystems, Inc.//DTD JSP Tag Library 1.1//EN" "http://java.sun.com/j2ee/dtds/web-jsptaglibrary_1_1.dtd"> <! a tag library descriptor > <taglib> <! after this the default space is "http://java.sun.com/j2ee/dtds/jsptaglibrary_1_2.dtd" > <tlibversion>1.0</tlibversion> <jspversion>1.1</jspversion> <shortname>jpptaglib</shortname> <info> A tag library from Java Primer Plus </info> <tag> <name>stringTag</name> <tagclass>com.samspublishing.jpp.ch25.StringTag</tagclass> <info>a Hello World custom tag example</info> <bodycontent>EMPTY</bodycontent> </tag> </taglib> The lines preceding <shortname> tag will be the same for every .tlb file that you create. This file is an XML file, so the version of XML used, as well as the Document Type Definition(DTD), for this file type are listed. See Chapter 25, "XML," for a more complete discussion of XML particulars. <?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE taglib PUBLIC "-//Sun Microsystems, Inc.//DTD JSP Tag Library 1.1//EN" "http://java.sun.com/j2ee/dtds/web-jsptaglibrary_1_1.dtd"> Following this, the version of the taglib and JSP are listed. <tlibversion>1.0</tlibversion> <jspversion>1.1</jspversion> Following that, a short name for this taglib is declared. <shortname>jpptaglib</shortname> The real work of this .tlb is found between the <tag> and </tag> tabs. <tag> The name of the custom tag is the name that it will be known by inside JSPs. <name>stringTag</name> The tagclass is the name of the class in the classpath of the Web server. This includes the package in which it is located. <tagclass>com.samspublishing.jpp.ch25.StringTag</tagclass> Info tags provide information to the reader in a manner similar to comments. <info>a Hello World custom tag example</info> The bodycontent tag will be used later when we create a more full-featured tag. <bodycontent>EMPTY</bodycontent> </tag> Now that we have a tag defined, we need a Java class to provide its implementation. This is the class that was named previously in the tagclass tag. Listing 24.2 shows this class. Listing 24.2 The StringTag.java File /* * StringTag.java * * Created on July 13, 2002, 12:38 PM */ package com.samspublishing.jpp.ch25; import javax.servlet.jsp.*; import javax.servlet.jsp.tagext.*; import java.io.*; /** * * @author Stephen Potts * @version */ public class StringTag extends TagSupport { public int doStartTag() { try { JspWriter out = pageContext.getOut(); out.print("Hello, String tag example "); }catch (Exception e) { System.out.println("Error in StringTag class" + e); } return(SKIP_BODY); } } Notice that the package name is the first item in the listing. This package name must match the directory structure under the rules of Java. package com.samspublishing.jpp.ch25; There are several import files required. import javax.servlet.jsp.*; import javax.servlet.jsp.tagext.*; import java.io.*; This class extends TagSupport, which implements the javax.servlet.jsp.tagext.Tag interface for us. public class StringTag extends TagSupport The doStartTag() method must be overridden to use the TagSupport class. This method is called automatically when the JSP engine is loading the custom tag into the page. public int doStartTag() { The only actual work that is done is performed here. A simple string is written out. JspWriter out = pageContext.getOut(); out.print("Hello, String tag example "); The return is a constant that tells the JSP there is no body to process. return(SKIP_BODY); Now the custom tag is ready to use. Listing 24.3 shows a JSP page that uses this tag. Listing 24.3 The StringTag.jsp File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN"> <html> <head> <%@ taglib uri="jpptaglib.tld" prefix="jpp" %> <title>A Simple Custom Tag</title> </head> <body> <h1>A Simple Custom Tag</h1> <br> <b><jpp:stringTag /> <b> </body> </html> This JSP file is ordinary in every respect with the exception of the use of the stringTag. The first step is to tell the JSP engine which taglib that you want to use. <%@ taglib uri="jpptaglib.tld" prefix="jpp" %> Now we can refer to the stringTag wherever we want. <b><jpp:stringTag /> <b> Whenever this string is encountered, the JSP engine runs the methods in the Java class. This is the class that was associated with this tag in the taglib file. The result is inserted in place and displayed along with the output from this JSP. To run this example, place both the JSP and the .tlb files in the same directory where you have been placing JSPs. Place the class file in the same directory where you have been placing class files, allowing for the package name, of course. On our test machine, these locations were as follows: Class file: install-dir\webapps\examples\WEB-INF\classes\com\samspublishing\jpp\ch25 JSP and .tlb: Install-dir\webapps\examples\jsp\jpp Figure 24.2 shows the result of running this JSP. Figure 24.2. The tag is translated at runtime, and the results are included in the response. 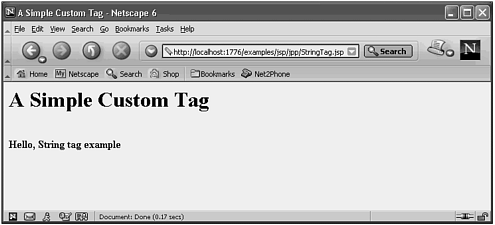 This example uses custom tags to do something that could easily be done without them, but it does illustrate the plumbing that is needed to get these tags to work. |