The Java servlet API gives us a way to manage state on the server side through the use of the HttpSession interface. This interface contains the usual dozen methods, but the most important ones are the constructor, getAttribute(), and setAttribute(). Internally, the Web server uses cookies to determine which of all the potentially active session objects is the one to provide to a particular servlet, but this processing is invisible to the programmer for security reasons. The basic flow of a session-oriented servlet is that one servlet will create the session object and place data in it. Subsequent calls to the Web server from that browser will have access to that stored data if they need it. Session objects differ from cookies in that they use the cookie to store only a browser identifier. All the other data is stored in the Web server. This means that the data will not be available if the Web server is restarted. If restarting could cause problems in your application, consider storing the data to a file or database periodically and reloading it when needed. We will modify the SpecialDiet servlet to use sessions instead of cookies and call it SpecialDietSession. This servlet is shown in Listing 21.17. Listing 21.17 The SpecialDietSession Servlet /* * SpecialDietSession.java * * Created on June 25, 2002, 1:27 PM */ import javax.servlet.*; import javax.servlet.http.*; import java.util.*; import java.io.*; /** * * @author Stephen Potts * @version */ public class SpecialDietSession extends HttpServlet { /** Initializes the servlet. */ public void init(ServletConfig config) throws ServletException { super.init(config); } /** Destroys the servlet. */ public void destroy() { } /** Handles the HTTP <code>GET</code> method. * @param request servlet request * @param response servlet response */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { } /** Handles the HTTP <code>POST</code> method. * @param request servlet request * @param response servlet response */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { //Get the Session Object HttpSession httpSess = request.getSession(true); Integer numItems = (Integer) httpSess.getAttribute("numItems"); if (numItems== null) { numItems = new Integer(0); } response.setContentType("text/html"); PrintWriter out = response.getWriter(); Enumeration keys; String key, value; keys = request.getParameterNames(); while (keys.hasMoreElements()) { key = (String)keys.nextElement(); value = request.getParameter(key); if(!key.equals("btnSubmit")) { System.out.println("SDS value= " + numItems + " " + value + " key= " + key); httpSess.setAttribute("Item" + numItems , key + " " + value); numItems = new Integer(numItems.intValue()+ 1); } } httpSess.setAttribute("numItems", numItems); // output your page here out.println("<html>"); out.println("<head>"); out.println("<title>" + "SpecialDietSession" + "</title>"); out.println("</head>"); out.println("<body BGCOLOR=\"#FDF5E6\"\n>"); out.println("<h1 ALIGN=CENTER>"); out.println("Session Object Populated"); out.println("<CENTER>"); out.println("<BR><BR>"); out.println("<A href=[ic:ccc] '/examples/servlet/SpecialDietSession2'>View Your Choices</A>"); out.println("</CENTER>"); out.println("</body>"); out.println("</html>"); out.close(); } /** Returns a short description of the servlet. */ public String getServletInfo() { return "Short description"; } } This servlet is fairly normal with the exception of the HttpSession processing. We use the HttpServletRequest object to get a handle to the HttpSession object. The Boolean value in the parameter to the getSession() method call states that a new session object is to be created if none exists for this client. HttpSession httpSess = request.getSession(true); We declare an attribute that will be used to track the number of attributes in the session object. Integer numItems = (Integer) httpSess.getAttribute("numItems"); We use an enumeration to process all the parameters in the request object. Enumeration keys; String key, value; keys = request.getParameterNames(); while (keys.hasMoreElements()) { key = (String)keys.nextElement(); value = request.getParameter(key); We want to store every parameter except btnSubmit. if(!key.equals("btnSubmit")) { System.out.println("SDS value= " + numItems + " " + value + " key= " + key); The setAttribute() method is the one that adds the parameters to the session object. We are adding an artificial name for each key-value pair called Itemx, where x is an increasing number. This is for convenience when retrieving all the attributes later. httpSess.setAttribute("Item" + numItems , key + " " + value); We increment a counter that we will store after the loop is complete. numItems = new Integer(numItems.intValue()+ 1); } } httpSess.setAttribute("numItems", numItems); The servlet is called by the ChooseDietSession.html file, which is shown in Listing 21.18. Listing 21.18 The ChooseDietSession.html File <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <HTML> <HEAD> <TITLE>Choose Your Dietary Preference</TITLE> </HEAD> <BODY BGCOLOR="#FDF5E6"> <H1 align="CENTER"> Do you have special dietary needs?: </H1> <FORM action='/examples/servlet/SpecialDietSession' method='post'> <TABLE cellspacing='5' cellpadding='5'> <TR> <TD align='center'><B>Choose one or more</B></TD> <TD align='center'><B></B></TD> <TD align='center'><B></B></TD> </TR> <TR> <TD align='center'><INPUT TYPE='Checkbox' NAME="losodium" VALUE="loso117"></TD> <TD align='center'>Delicious Low-Sodium Pizza</TD> </TR> <TR> <TD align='center'><INPUT TYPE='Checkbox' NAME="lofat" VALUE="lofat118"></TD> <TD align='center'>Delicious Lo-Fat Chicken</TD> </TR> <TR> <TD align='center'><INPUT TYPE='Checkbox' NAME="vegan" VALUE="vegan119"></TD> <TD align='center'>Delicious Vegetarian Lasagna</TD> </TR> </TABLE> <HR><BR> <CENTER> <INPUT type='SUBMIT' name='btnSubmit' value='OK'> <BR><BR> <A href='/examples/servlet/SpecialDietSession2'>View Current Choices</A> </CENTER> </FORM> </BODY> </HTML> Figure 21.13 shows what the ChooseDietSession.html file looks like when displayed. Figure 21.13. The ChooseDietSession HTML file gives the user the choices and calls SpecialDietSession. 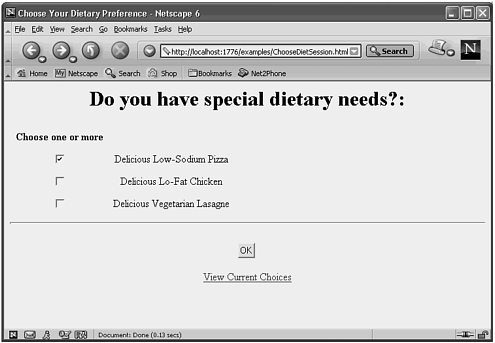 At the end of the SpecialDietSession servlet, a message is displayed back to the user that contains a hyperlink to the SpecialDietSession2 servlet. out.println( "<A href='/examples/servlet/SpecialDietSession2'>View Your Choices</A>"); Listing 21.19 shows this display-oriented servlet. After the OK button is clicked, you will be taken to a confirmation screen as shown in Figure 21.14. Figure 21.14. The SpecialDietSession servlet displays a confirmation page that contains a hyperlink to view the results. 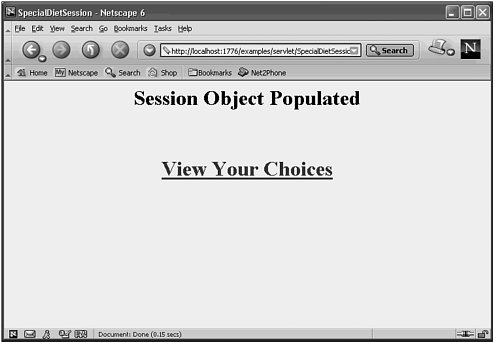 Listing 21.19 The SpecialDietSession2 Servlet /* * SpecialDietSession2.java * * Created on June 25, 2002, 1:27 PM */ import javax.servlet.*; import javax.servlet.http.*; import java.util.*; import java.io.*; /** * * @author Stephen Potts * @version */ public class SpecialDietSession2 extends HttpServlet { /** Initializes the servlet. */ public void init(ServletConfig config) throws ServletException { super.init(config); } /** Destroys the servlet. */ public void destroy() { } /** Handles the HTTP <code>GET</code> method. * @param request servlet request * @param response servlet response */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { HttpSession httpSess = request.getSession(true); response.setContentType("text/html"); PrintWriter out = response.getWriter(); System.out.println("Creating the SpecialDiet HTML"); // output your page here out.println("<html>"); out.println("<head>"); out.println("<title>" + "Your Diet Choices" + "</title>"); out.println("</head>"); out.println("<body BGCOLOR=\"#FDF5E6\"\n>"); out.println("<h1 ALIGN=CENTER>"); out.println("Here are your choices"); out.println("</h1>"); Integer numItems = (Integer) httpSess.getAttribute("numItems"); for (int i=0; i< numItems.intValue(); i++) { String itemContents = (String)httpSess.getAttribute("Item" + i); System.out.println(itemContents + "<BR>"); out.println("Item" + i + "= " + itemContents + "<BR>"); out.println(" "); } out.println("</body>"); out.println("</html>"); httpSess.invalidate(); out.close(); } /** Handles the HTTP <code>POST</code> method. * @param request servlet request * @param response servlet response */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, java.io.IOException { } /** Returns a short description of the servlet. */ public String getServletInfo() { return "Short description"; } } This servlet mostly displays code. Its main feature is that it steps through the entries of the session object and outputs them to the browser. Integer numItems = (Integer) httpSess.getAttribute("numItems"); for (int i=0; i< numItems.intValue(); i++) { String itemContents = (String)httpSess.getAttribute("Item" + i); System.out.println(itemContents + "<BR>"); out.println("Item" + i + "= " + itemContents + "<BR>"); out.println(" "); } The getAttribute() method is the main player here. The contents of the session object are referenced by name and printed. At the end, we invalidated the session object, which causes the information in it to be purged. If we didn't do this, the entries would continue to be added each time we ran the servlets, which is not the desired result. Figure 21.15 shows the result of running the SpecialDietSession2. Figure 21.15. The SpecialDietSession2 servlet displays the results. 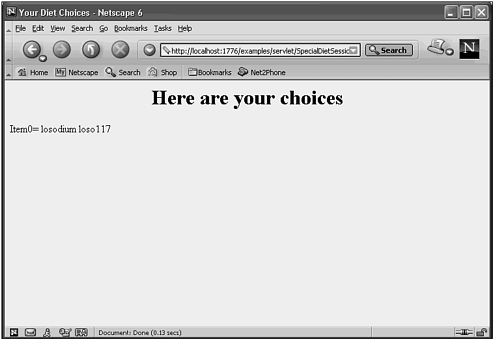 The contents of the session object are displayed both for you to inspect and to prove that they retained their values across different servlet calls. |