[Page 202 (continued)]6.7. Chromakey The way that weatherpersons appear to be in front of a weather map that changes, is that they actually stand before a background of a fixed color (usually blue or green), then subtract that color. This is called chromakey. Mark took our son's blue sheet, attached it to the entertainment center, then took a picture of himself in front of it, using a timer on a camera (Figure 6.19). Figure 6.19. Mark in front of a blue sheet. 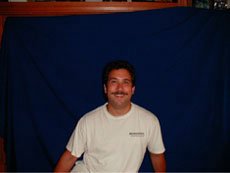 [Page 203]Mark tried a new way to test for "blueness." If the blue value was greater than the sum of the red and green values then the color was "blue." Program 45. Chromakey: Replace all Blue with the New Background /** * Method to do chromakey using a blue background * @param newBg the new background image to use to replace * the blue from the current picture */ public void chromakey(Picture newBg) { Pixel currPixel = null; Pixel newPixel = null; // loop through the columns for (int x=0; x<getWidth(); x++) { // loop through the rows for (int y=0; y<getHeight(); y++) { // get the current pixel currPixel = this.getPixel(x,y); /* if the color at the current pixel is mostly blue * (blue value is greater than red and green * combined), then use new background color */ if (currPixel.getRed() + currPixel.getGreen() < currPixel.getBlue()) { newPixel = newBg.getPixel(x,y); currPixel.setColor(newPixel.getColor()); } } } } | The effect is really quite striking (Figure 6.20). Do note the "folds" in the lunar surface, though. The really cool thing is that this program works for any background that's the same size as the image (Figure 6.21). To put Mark on the moon and on the beach, try this: > String fileName = FileChooser.getMediaPath("blue-mark.jpg"); > Picture mark = new Picture(fileName); > fileName = FileChooser.getMediaPath("moon-surface.jpg"); [Page 204]> Picture newBg = new Picture(fileName); > mark.chromakey(newBg); > mark.explore(); > mark = new Picture( FileChooser.getMediaPath("blue-mark.jpg")); > newBg = new Picture(FileChooser.getMediaPath("beach.jpg")); > mark.chromakey(newBg); > mark.explore(); Figure 6.20. Mark on the moon. 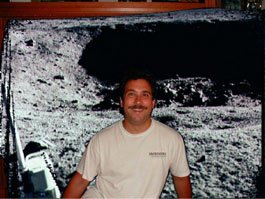 Figure 6.21. Mark on the beach. 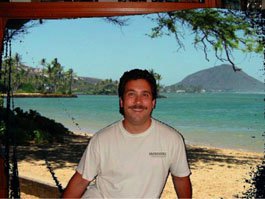 [Page 205]There's another way of writing this code, which is shorter but does the same thing. Program 46. Chromakey, Shorter /** * Method to do chromakey using a blue background * @param newBg the new background image to use to replace * the blue from the current picture */ public void chromakeyBlue(Picture newBg) { Pixel[] pixelArray = this.getPixels(); Pixel currPixel = null; Pixel newPixel = null; // loop through the pixels for (int i = 0; i < pixelArray.length; i++) { // get the current pixel currPixel = pixelArray[i]; /* if the color at the current pixel is mostly blue * (blue value is greater than green and red * combined), then use new background color */ if (currPixel.getRed() + currPixel.getGreen() < currPixel.getBlue()) { newPixel = newBg.getPixel(currPixel.getX(), currPixel.getY()); currPixel.setColor(newPixel.getColor()); } } } |  | Making it Work Tip: When Do You Need a Different Method Name?Notice that we used a different name for the new shorter method. We couldn't have used the same name and had both methods in our Picture class since the parameters are the same. Methods can be overloaded (use the same name) as long as the parameters are different (in number, or order, or type). |
|
You don't really want to do chromakey with a common color, like redsomething that there's a lot of in your face. If you do, some of the person's face will be swapped. This is why moviemakers and weather people use blue or green backgrounds. |