Take a look at the example in Figure 8.3, ButtonProject.java, which is designed to show how to handle events in SWT applications. When you click the button in this project, the application catches that event and then the message "Thanks for clicking." appears in the Text widget. Figure 8.3. Handling events in SWT applications. 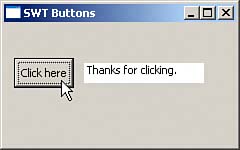
This application starts by creating the Button widget it needs, giving it the caption "Click here," and making it a push button (the allowed styles are SWT.ARROW, SWT.CHECK, SWT.PUSH, SWT.RADIO, SWT.TOGGLE, SWT.FLAT, SWT.UP, SWT.DOWN, SWT.LEFT, SWT.RIGHT, and SWT.CENTER): import org.eclipse.swt.*; import org.eclipse.swt.events.*; import org.eclipse.swt.widgets.*; public class ButtonProject { Display display; Shell shell; Button button; public static void main(String [] args) { new ButtonProject(); } ButtonProject() { display = new Display(); shell = new Shell(display); shell.setSize(240, 150); shell.setText("SWT Buttons"); button = new Button(shell, SWT.PUSH); button.setText("Click here"); button.setBounds(10, 35, 60, 30); . . . } You can find the significant methods of the SWT Button class in Table 8.3. Table 8.3. Significant Methods of the org.eclipse.swt.widgets.Button ClassMethod | Does This |
---|
void addSelectionListener (SelectionListener listener) | Adds the given listener to the listeners that will be notified when an event occurs in the Text widget | Point computeSize(int wHint, int hHint, boolean changed) | Returns the preferred size of the Text widget | int getAlignment() | Returns a constant indicating the position of text or an image in the Text widget | Image getImage() | Returns the Text widget's image, if it has one, or null otherwise | boolean getSelection() | Returns TRue if the Text widget is selected. Returns false otherwise | String getText() | Returns the Text widget's text, if there is any, or null otherwise | void removeSelectionListener (SelectionListener listener) | Removes the listener from the listeners that will be notified when the Text widget is selected by the user | void setAlignment(int alignment) | Sets how text, images, and arrows will be displayed in the Text widget | void setImage(Image image) | Sets the image displayed in the Text widget to the given image | void setSelection(boolean selected) | Sets the selection state of the Text widget | void setText(String string) | Sets the text that will appear in the Text widget |
To handle button clicks, you add a SelectionListener to the button, which you can do with the addSelectionListener method. Listeners in SWT are much like listeners in AWT; here are some of the most popular: Listener Handles generic events ControlListener Handles moving and resizing FocusListener Handles the getting and losing of focus KeyListener Handles keystrokes MouseListener, MouseMoveListener, and MouseTrackListener Handle the mouse SelectionListener Handles widget selections (including button clicks) You use a SelectionListener object to handle button clicks. To implement a selection listener, you need to override two methods: widgetSelected, called when the widget is selected or clicked, and widgetDefaultSelected, called when the default event for the window occurs (such as when the user enters text in a Text widget and presses Enter). Here's how to add the target Text widget and handle the button-click event in the ButtonProject application (the allowed styles are SWT.CENTER, SWT.LEFT, SWT.MULTI, SWT.PASSWORD, SWT.SINGLE, SWT.RIGHT, SWT.READ_ONLY, SWT.WRAP, SWT.SHADOW_IN, and SWT.SHADOW_OUT): import org.eclipse.swt.*; import org.eclipse.swt.events.*; import org.eclipse.swt.widgets.*; public class ButtonProject { Display display; Shell shell; Button button; Text text; public static void main(String [] args) { new ButtonProject(); } ButtonProject() { . . . button = new Button(shell, SWT.PUSH); button.setText("Click here"); button.setBounds(10, 35, 60, 30); text = new Text(shell, SWT.SHADOW_IN); text.setBounds(80, 40, 120, 20); button.addSelectionListener(new SelectionListener() { public void widgetSelected(SelectionEvent event) { text.setText("Thanks for clicking."); } public void widgetDefaultSelected(SelectionEvent event) { text.setText("No worries!"); } }); shell.open(); while(!shell.isDisposed()) { if(!display.readAndDispatch()) display.sleep(); } display.dispose(); } } You can find the significant methods of the SWT Text class, such as setText to set the text in the widget, in Table 8.4. Table 8.4. Significant Methods of the org.eclipse.swt.widgets.Text ClassMethod | Does This |
---|
void addSelectionListener (SelectionListener listener) | Adds the given selection listener to the listeners that will be notified when the Text widget is selected | void addModifyListener (ModifyListener listener) | Adds the given modify listener to the listeners that will be notified when the Text widget is selected | void addVerifyListener (VerifyListener listener) | Adds the given verify listener to the listeners that will be notified when the Text widget is selected | void append(String string) void clearSelection() Point computeSize(int wHint, int hHint, boolean changed) | Appends a string to the text in the Text widget Clears the current selection in the Text widget Returns the preferred size of the Text widget | void copy() | Copies the text currently selected in the Text widget | void cut() | Cuts the text currently selected in the Text widget | int getBorderWidth() | Returns the width of the Text widget's border | boolean getEditable() | Returns TRue if the text in the Text widget may be edited | int getLineCount() | Returns the number of lines in the Text widget | int getLineHeight() | Returns the height of a line in the Text widget | int getOrientation() | Returns the current orientation of the Text widget, which can be either SWT.LEFT_TO_RIGHT or SWT.RIGHT_TO_LEFT | Point getSelection() | Returns the location of the selected text in the Text widget | int getSelectionCount() | Returns the number of characters selected in the Text widget | String getSelectionText() | Returns the text currently selected in the Text widget | String getText() | Returns the text in the Text widget | String getText(int start, int end) | Returns a range of text, extending from start to end | int getTextLimit() | Returns the maximum number of characters that the Text widget can contain | void insert(String string) | Inserts a text string into the Text widget | void paste() | Pastes text from the clipboard into the Text widget | void removeModifyListener (ModifyListener listener) | Removes the given modify listener from the Text widget | void removeSelectionListener (SelectionListener listener) | Removes the given selection listener from the Text widget | void removeVerifyListener (VerifyListener listener) | Removes the given verify listener from the Text widget | void selectAll() | Selects all the text in the Text widget, making it the current selection | void setEditable(boolean editable) | Sets whether the text in the Text widget may be edited | void setFont(Font font) | Sets the font that will be used in the Text widget for text | void setOrientation(int orientation) | Sets the orientation of the Text widget: SWT.LEFT_TO_RIGHT or SWT.RIGHT_TO_LEFT | void setSelection(int start) | Sets the current selection in the Text widget, from the start to the end of the text | void setSelection(int start, int end) | Sets the current selection in the Text widget, from start to end | void setText(String string) | Sets the text in the Text widget to the specified text |
You can see the results in Figure 8.3; when you click the button, the application's message appears in the Text widget as it should. Very nice. |