Formatting and parsing, as defined by JSTL, are two related but quite different actions. JSTL considers formatting to be the process of changing the appearance of stored data. This data has already been validated to be correct, and most likely should not produce an error. JSTL provides two tags for data formatting. The <fmt:formatNumber> tag is used to format numbers; the <fmt:formatDate> tag is used to format dates and times. Using the <fmt:formatNumber> Tag The <fmt:formatNumber> tag is used to format numbers, percentages, and currencies. There are two forms of this tag: // Syntax 1: Without a body <fmt:formatNumber value="numericValue" [type="{number|currency|percent}"] [pattern="customPattern"] [currencyCode="currencyCode"] [currencySymbol="currencySymbol"] [groupingUsed="{true|false}"] [maxIntegerDigits="maxIntegerDigits"] [minIntegerDigits="minIntegerDigits"] [maxFractionDigits="maxFractionDigits"] [minFractionDigits="minFractionDigits"] [var="varName"] [scope="{page|request|session|application}"]/> // Syntax 2: With a body to specify the numeric value to be formatted <fmt:formatNumber [type="{number|currency|percent}"] [pattern="customPattern"] [currencyCode="currencyCode"] [currencySymbol="currencySymbol"] [groupingUsed="{true|false}"] [maxIntegerDigits="maxIntegerDigits"] [minIntegerDigits="minIntegerDigits"] [maxFractionDigits="maxFractionDigits"] [minFractionDigits="minFractionDigits"] [var="varName"] [scope="{page|request|session|application}"]> numeric value to be formatted </fmt:formatNumber> The attributes accepted by the <fmt:formatNumber> tag include the following: currencyCode | N | String ISO 4217 currency code. Applied only when formatting currencies (that is, if type is equal to currency); ignored otherwise. | currencySymbol | N | String currency symbol. Applied only when formatting currencies (that is, if type is equal to currency); ignored otherwise. | groupingUsed | N | Specifies whether the formatted output will contain any grouping separators. The default value is true. | maxFractionDigits | N | The maximum number of digits in the fractional portion of the formatted output. | maxIntegerDigits | N | The maximum number of digits in the integer portion of the formatted output. | minFractionDigits | N | The minimum number of digits in the fractional portion of the formatted output. | minIntegerDigits | N | The minimum number of digits in the integer portion of the formatted output. | pattern | Y | The string custom formatting pattern. Applied only when formatting numbers (that is, if type is missing or is equal to number); ignored otherwise. | scope | N | The scope of var. The default value is page. | type | N | Specifies whether the value is to be formatted as a number, currency, or percentage. The default value is number. | value | N | The string or number numeric value to be formatted. | var | N | The name of the exported scoped attribute that stores the formatted result as a string. | In the first syntax, the value to be formatted is passed in using the value attribute. The formatted number will be written to the page if a var attribute is not specified. If a var attribute is specified, it will receive the formatted number as a string. The second syntax uses the body of the tag to specify the number that is to be formatted. You should not provide a value attribute if you are using the tag with a body (syntax 2, in our example). In addition to these standard JSTL attributes, several formatting attributes are available specific to number formatting. A type attribute is provided to specify what type of number this is. Valid values for the type attribute are number, currency, and percent. If the type attribute is currency, then the currencyCode and currencySymbol attributes can also be specified. The currencyCode attribute specifies an ISO4217 currency code for this number. This allows JSTL to know what type of currency you are providing. You can also override the default currency symbol for the specified currency by using the currencySymbol attribute. NOTE You can obtain ISO4217, for a fee, from the ISO Web site (http://www.iso.ch/). You can also find a Microsoft Word document containing these codes at http://www.bsi-global.com/Technical+Information/Publications/_Publications/tig90.xalter. This site is the official ISO Web repository for the ISO4217 codes. If the type attribute is percent or number, then you can use several number-formatting attributes. The maxIntegerDigits and minIntegerDigits attributes allow you to specify the size of the nonfractional portion of the number. If the actual number exceeds maxIntegerDigits, then the number is truncated. Attributes are also provided to allow you to determine how many decimal places should be used. The minFractionalDigits and maxFractionalDigits attributes allow you to specify the number of decimal places. If the number exceeds the maximum number of fractional digits, the number will be rounded. Grouping can be used to insert commas between thousands groups. Grouping is specified by setting the groupingIsUsed attribute to either true or false. When using grouping with minIntegerDigits, you must be careful to get your intended result. For example, if the value 1000 were instructed to have a minimum of 8 digits and grouping is used, the result would be 00,0001,000 If the previously mentioned formatting attributes are insufficient, you may elect to use the pattern attribute. This attribute lets you include special characters that specify how you would like your number encoded. Table 6.1 shows these codes. Table 6.1. Numeric Formatting Codes 0 | Represents a digit. | # | Represents a digit; displays 0 as absent. | . | Serves as a placeholder for a decimal separator. | , | Serves as a placeholder for a grouping separator. | ; | Separates formats. | - | Used as the default negative prefix. | % | Multiplies by 100 and displays as a percentage. | ? | Multiplies by 1000 and displays as per mille. | | Represents the currency sign; replaced by actional currency symbol. | X | Indicates that any other characters can be used in the prefix or suffix. | ' | Used to quote special characters in a prefix or suffix. | Now that you have seen how to use the <fmt:formatNumber> tag, let's look at an example that uses this tag. Listing 6.1 shows a simple program that will format numbers. The program allows you to enter numbers and see them formatted in a variety of ways by JSTL. Figure 6.1 shows the output of our program. Listing 6.1 Formatting Numbers (string.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <%@ taglib uri="http://java.sun.com/jstl/fmt" prefix="fmt" %> <html> <head> <title>Format Number</title> </head> <body> <form method="POST"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="62%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Number Formatting</font> </b> </p> </td> </tr> <tr> <td width="47%">Enter a number to be formatted:</td> <td width="53%"> <input type="text" name="num" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="Submit" name="submit" /> <input type="reset" value="Reset" name="reset" /> </p> </td> </tr> </table> <p> </p> </form> <c:if test="${pageContext.request.method=='POST'}"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="63%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Formatting: <c:out value="${param.num}" /> </font> </b> </p> </td> </tr> <tr> <td width="51%">type="number"</td> <td width="49%"> <fmt:formatNumber type="number" value="${param.num}" /> </td> </tr> <tr> <td>type="number" maxIntegerDigits="3"</td> <td> <fmt:formatNumber type="number" maxIntegerDigits="3" value="${param.num}" /> </td> </tr> <tr> <td>type="number" minIntegerDigits="10"</td> <td> <fmt:formatNumber type="number" minIntegerDigits="10" value="${param.num}" /> </td> </tr> <tr> <td>type="number" maxFractionDigits="3"</td> <td> <fmt:formatNumber type="number" maxFractionDigits="3" value="${param.num}" /> </td> </tr> <tr> <td>type="number" minFractionDigits="10"</td> <td> <fmt:formatNumber type="number" minFractionDigits="10" value="${param.num}" /> </td> </tr> <tr> <td>type="number" maxFractionDigits="3" groupingUsed="false"</td> <td> <fmt:formatNumber type="number" maxFractionDigits="3" groupingUsed="false" value="${param.num}" /> </td> </tr> </table> </c:if> </body> </html> 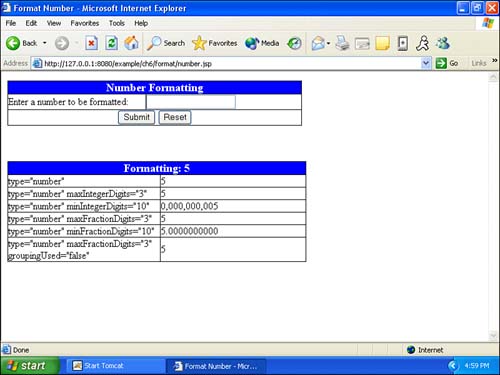 This program displays the number the user entered using variations of this <fmt:formatNumber> tag: <fmt:formatNumber type="number" maxFractionDigits="3" groupingUsed="false" value="${param.num}" /> It is possible to throw an exception using the <fmt:formatNumber> tag. If you enter a text value such as one, the program will throw an exception. If you would like to prevent such exceptions from stopping your program, you must use the <c:catch> tag that we discussed in Chapter 4, "Using the Expression Language." When you use very large numbers, rounding will sometimes occur, even though you have maxFractionDigits set to a large enough value to not require rounding. This odd behavior seems to occur only with very large numbers. Additionally, there appears to be an undocumented default of 3 for maxFractionDigits. Formatting Percents You can also format percents by using <fmt:formatNumber>. To format a percent, you must set the type attribute to percent. Numbers that are to be formatted as percent must be expressed in the conventional ratio form (for example, 50% is represented as .5). Every other numeric formatting option that we described in the previous section also works with percent numbers. Listing 6.2 shows percent formatting applied to the same numeric formats used in our earlier example. Figure 6.2 shows the output from this program. As you can see, the percent symbol (%) is added. Whatever percentage indicator specified for the current locale will be used. Listing 6.2 Formatting Percents (percent.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <%@ taglib uri="http://java.sun.com/jstl/fmt" prefix="fmt" %> <html> <head> <title>Format Percent</title> </head> <body> <form method="POST"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="62%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Number Formatting</font> </b> </p> </td> </tr> <tr> <td width="47%">Enter a percent to be formatted:</td> <td width="53%"> <input type="text" name="num" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="Submit" name="submit" /> <input type="reset" value="Reset" name="reset" /> </p> </td> </tr> </table> <p> </p> </form> <c:if test="${pageContext.request.method=='POST'}"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="63%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Formatting: <c:out value="${param.num}" /> </font> </b> </p> </td> </tr> <tr> <td width="51%">type="percent"</td> <td width="49%"> <fmt:formatNumber type="percent" value="${param.num}" /> </td> </tr> <tr> <td>type="percent" maxIntegerDigits="3"</td> <td> <fmt:formatNumber type="percent" maxIntegerDigits="3" value="${param.num}" /> </td> </tr> <tr> <td>type="percent" minIntegerDigits="10"</td> <td> <fmt:formatNumber type="percent" minIntegerDigits="10" value="${param.num}" /> </td> </tr> <tr> <td>type="percent" maxFractionDigits="3"</td> <td> <fmt:formatNumber type="percent" maxFractionDigits="3" value="${param.num}" /> </td> </tr> <tr> <td>type="percent" minFractionDigits="10"</td> <td> <fmt:formatNumber type="percent" minFractionDigits="10" value="${param.num}" /> </td> </tr> <tr> <td>type="percent" maxFractionDigits="3" groupingUsed="false"</td> <td> <fmt:formatNumber type="percent" maxFractionDigits="3" groupingUsed="false" value="${param.num}" /> </td> </tr> </table> </c:if> </body> </html> 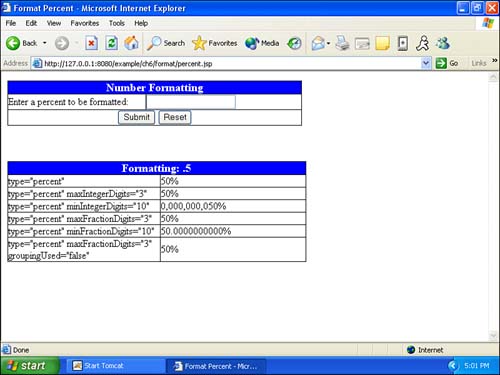 Using the <fmt:formatDate> Tag There are many occasions when a program must display dates. There are also many formats that a date can be displayed in. By using the <fmt:formatDate> tag, you can express your program's dates in a variety of ways. The <fmt:formatDate> tag is shown here: <fmt:formatDate [value="date"] [type="{time|date|both}"] [dateStyle="{default|short|medium|long|full}"] [timeStyle="{default|short|medium|long|full}"] [pattern="customPattern"] [timeZone="timeZone"] [var="varName"] [scope="{page|request|session|application}"]/> The attributes that are accepted by the <fmt:formatDate> tag include the following: dateStyle | N | The predefined formatting style for dates. Applied only when formatting a date or both a date and time (that is, if type is missing or is equal to date or both); ignored otherwise. This attribute uses DateFormat semantics. | pattern | N | The custom formatting style for dates and times. | scope | N | The scope of var. | timeStyle | N | The predefined formatting style for times. Applied only when formatting a time or both a date and time (that is, if type is missing or is equal to date or both); ignored otherwise. | timeZone | N | The time zone in which you want to represent the formatted time. | type | N | Specifies whether only the time, the date, or both the time and date components of the given date are to be formatted. This attribute defaults to date. | value | Y | The date and/or time to be formatted. | var | N | The name of the exported scoped attribute that stores the formatted result as a string. This attribute defaults to page. | In the first syntax, the value that is to be formatted is passed in using the value attribute. The formatted date will be written to the page if a var attribute is not specified. If a var attribute is specified, it will receive the formatted date as a string. The second syntax uses the body of the tag to specify the date that is to be formatted. You should not provide a value attribute if you are using the tag with a body (syntax 2 in our example). In addition to these standard JSTL attributes, several formatting attributes are available specific to date formatting. The type attribute allows you to specify whether this is a time, date, or both. The valid values for the type attribute are time, date, or both; date is the default. Additionally, you can specify the time and date styles using the timeStyle and dateStyle attributes. Both of these accept the values default, short, medium, long, or full. These values specify the amount of detail that will be displayed. It is also possible to use the pattern attribute to specify even more precise handling of the date formatting. Table 6.2 lists the format characters that can be used. Table 6.2. Date-Formatting Codes G | The era designator | AD | y | The year | 2002 | M | The month | April & 04 | d | The day of the month | 20 | h | The hour(12-hour time) | 12 | H | The hour(24-hour time) | 0 | m | The minute | 45 | s | The second | 52 | S | The millisecond | 970 | E | The day of the week | Tuesday | D | The day of the year | 180 | F | The day of the week in the month | 2 (2nd Wed in month) | w | The week in the year | 27 | W | The week in the month | 2 | a | The a.m./p.m. indicator | PM | k | The hour(12-hour time) | 24 | K | The hour(24-hour time) | 0 | z | The time zone | Central Standard Time | ' | | The escape for text | '' | | The single quote | Using these codes gives you precise control of the output. For example, using the pattern string of hh 'o''clock' a, zzzz, you might get back 2 o'clock PM, Central Daylight Time. To get an idea of what some of these formats look like, let's examine Listing 6.3. The output from this program can be seen in Figure 6.3. Listing 6.3 Formatting Dates (date.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <%@ taglib uri="http://java.sun.com/jstl/core-rt" prefix="c-rt" %> <%@ taglib uri="http://java.sun.com/jstl/fmt" prefix="fmt" %> <html> <head> <title>Format Date</title> </head> <body> <c-rt:set var="now" value="<%=new java.util.Date()%>" /> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="63%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font color="#FFFFFF" size="4">Formatting: <fmt:formatDate value="${now}" type="both" timeStyle="long" dateStyle="long" /> </font> </b> </p> </td> </tr> <tr> <td width="51%">formatDate type="time"</td> <td width="49%"> <fmt:formatDate type="time" value="${now}" /> </td> </tr> <tr> <td width="51%">type="date"</td> <td width="49%"> <fmt:formatDate type="date" value="${now}" /> </td> </tr> <tr> <td width="51%">type="both"</td> <td width="49%"> <fmt:formatDate type="both" value="${now}" /> </td> </tr> <tr> <td width="51%">type="both" dateStyle="default" timeStyle="default"</td> <td width="49%"> <fmt:formatDate type="both" dateStyle="default" timeStyle="default" value="${now}" /> </td> </tr> <tr> <td width="51%">type="both" dateStyle="short" timeStyle="short"</td> <td width="49%"> <fmt:formatDate type="both" dateStyle="short" timeStyle="short" value="${now}" /> </td> </tr> <tr> <td width="51%">type="both" dateStyle="medium" timeStyle="medium"</td> <td width="49%"> <fmt:formatDate type="both" dateStyle="medium" timeStyle="medium" value="${now}" /> </td> </tr> <tr> <td width="51%">type="both" dateStyle="long" timeStyle="long"</td> <td width="49%"> <fmt:formatDate type="both" dateStyle="long" timeStyle="long" value="${now}" /> </td> </tr> <tr> <td width="51%">type="both" dateStyle="full" timeStyle="full"</td> <td width="49%"> <fmt:formatDate type="both" dateStyle="full" timeStyle="full" value="${now}" /> </td> </tr> <tr> <td width="51%">pattern="yyyy-MM-dd"</td> <td width="49%"> <fmt:formatDate pattern="yyyy-MM-dd" value="${now}" /> </td> </tr> </table> </body> </html> 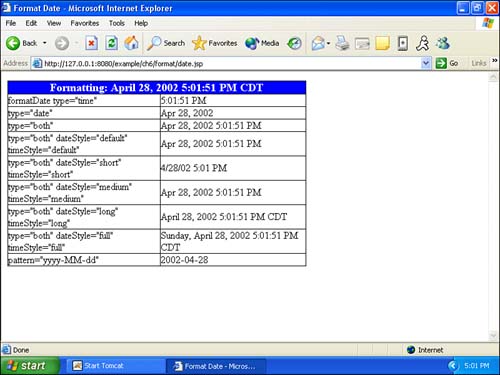 The program in Listing 6.3 begins by obtaining the current date. This can be done with the following RT tag: <c-rt:set var="now" value="<%=new java.util.Date()%>" /> This tag constructs a new Date object, which will contain the current time. This date is then assigned to the scoped variable now. Now that the date has been obtained, the program can display the date with a variety of <fmt:formatDate> tags. |