Working with various fonts has never been a trivial task. Each font has specific characteristics such as its height, width, ascent, and decent. Fortunately, working with the Font class provided by the base class libraries makes working with fonts a painless task. Fonts, like brushes and pens, can be solid or textured and can be any size or color. Included with the .NET Framework SDK is a wonderful font example that includes textured fonts, drop shadows, internalization, and font rotation. For the sake of completeness, the Font Demo application will be shown to illustrate the following topics: Drop shadows Textured fonts Background images Graphics transformations The Font Demo will be in the style of the Pen Demo presented earlier in this chapter, enabling the property grid to be used to manipulate the various properties and options. Figure 4.6 shows the Font Demo application with the source given in Listing 4.7. Figure 4.6. The Font Demo.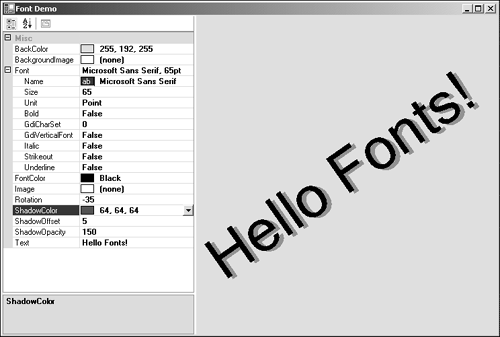 Listing 4.7 Font Demo Source 1: using System; 2: using System.Drawing; 3: using System.Drawing.Drawing2D; 4: using System.Collections; 5: using System.ComponentModel; 6: using System.Windows.Forms; 7: using System.Data; 8: 9: namespace FontDemo 10: { 11: 12: public class FontProperties { 13: private Font theFont; 14: private Color fontColor; 15: private Brush theFontBrush; 16: private Image theImage; 17: 18: private Color backgroundColor; 19: private Image backgroundImage; 20: private Brush backgroundBrush; 21: 22: private Color shadowColor; 23: private Brush theShadowBrush; 24: private int shadowOffset; 25: private int shadowOpacity; 26: private float rotation; 27: 28: private string text; 29: 30: public event EventHandler PropertyChanged; 31: 32: 33: public Font Font { 34: get { return theFont; } 35: set { 36: theFont = value; 37: OnPropertyChanged( new EventArgs( ) ); 38: } 39: } 40: 41: public Color FontColor { 42: get { return fontColor; } 43: set { 44: fontColor = value; 45: theFontBrush = new SolidBrush( fontColor ); 46: OnPropertyChanged( new EventArgs( ) ); 47: } 48: } 49: 50: public Color ShadowColor { 51: get { return shadowColor; } 52: set { 53: shadowColor = value; 54: theShadowBrush = new SolidBrush( Color.FromArgb( shadowOpacity, shadowColor ) ); 55: OnPropertyChanged( new EventArgs( ) ); 56: } 57: } 58: 59: public int ShadowOpacity { 60: get { return shadowOpacity; } 61: set { 62: shadowOpacity = value; 63: theShadowBrush = new SolidBrush( Color.FromArgb( shadowOpacity, shadowColor ) ); 64: OnPropertyChanged( new EventArgs( ) ); 65: } 66: } 67: 68: public int ShadowOffset { 69: get { return shadowOffset; } 70: set { 71: shadowOffset = value; 72: OnPropertyChanged( new EventArgs( ) ); 73: } 74: } 75: public Image Image { 76: get { return theImage; } 77: set { 78: theImage = value; 79: theFontBrush = new TextureBrush( theImage ); 80: OnPropertyChanged( new EventArgs( ) ); 81: } 82: } 83: 84: public Color BackColor { 85: get { return backgroundColor; } 86: set { 87: backgroundColor = value; 88: backgroundBrush = new SolidBrush( backgroundColor ); 89: OnPropertyChanged( new EventArgs( ) ); 90: } 91: } 92: 93: public Image BackgroundImage { 94: get { return backgroundImage; } 95: set { 96: backgroundImage = value; 97: if( backgroundImage != null ) 98: backgroundBrush = new TextureBrush(backgroundImage); 99: else 100: backgroundBrush = new SolidBrush( backgroundColor ); 101: OnPropertyChanged( new EventArgs( ) ); 102: } 103: } 104: 105: public string Text { 106: get { return text; } 107: set { 108: text = value; 109: OnPropertyChanged( new EventArgs( ) ); 110: } 111: } 112: 113: public float Rotation { 114: get { return rotation; } 115: set { 116: rotation = value; 117: OnPropertyChanged( new EventArgs( ) ); 118: } 119: } 120: 121: 122: //Properties to be used by Font Demo 123: //The BrowsableAttribute is used by the Property 124: //Grid to determine if the property should be 125: //accessable or not. 126: 127: [System.ComponentModel.Browsable( false )] 128: public Brush FontBrush { 129: get { return theFontBrush; } 130: set { theFontBrush = value; } 131: } 132: 133: [System.ComponentModel.Browsable( false )] 134: public Brush ShadowBrush { 135: get { return theShadowBrush; } 136: set { theShadowBrush = value; } 137: } 138: 139: [System.ComponentModel.Browsable( false )] 140: public Brush BackgroundBrush { 141: get { return backgroundBrush; } 142: set { backgroundBrush = value; } 143: } 144: 145: protected void OnPropertyChanged( EventArgs e ) { 146: if( PropertyChanged != null ) 147: PropertyChanged( this, e ); 148: } 149: } 150: 151: 152: 153: 154: 155: public class Form1 : System.Windows.Forms.Form 156: { 157: private FontProperties fontProperties; 158: private System.Windows.Forms.PropertyGrid propertyGrid1; 159: private System.Windows.Forms.Splitter splitter1; 160: /// <summary> 161: /// Required designer variable. 162: /// </summary> 163: private System.ComponentModel.Container components = null; 164: 165: 166: public Form1() 167: { 168: // 169: // Required for Windows Form Designer support 170: // 171: InitializeComponent(); 172: 173: this.SetStyle( ControlStyles.ResizeRedraw, true ); 174: 175: fontProperties = new FontProperties( ); 176: 177: //set default values 178: fontProperties.Font = (Font)this.Font.Clone( ); 179: fontProperties.FontColor = Color.Black; 180: fontProperties.ShadowColor = Color.LightGray; 181: fontProperties.ShadowOpacity = 100; //range 0 to 255 182: fontProperties.BackgroundBrush = new SolidBrush(Color.White); 183: fontProperties.Text = "Hello Fonts!"; 184: fontProperties.PropertyChanged += new EventHandler( this.OnFPPropertyChangedEventHandler ); 185: propertyGrid1.SelectedObject = fontProperties; 186: propertyGrid1.Refresh( ); 187: 188: 189: this.Invalidate( ); 190: 191: } 192: 193: 194: 195: protected void OnFPPropertyChangedEventHandler( object sender, EventArgs e ) { 196: this.Invalidate( ); 197: } 198: 199: protected override void OnPaint( PaintEventArgs e ) { 200: Rectangle drawZone = new Rectangle( DisplayRectangle.Left + propertyGrid1.Width, 201: DisplayRectangle.Top, 202: DisplayRectangle.Width propertyGrid1.Width, 203: DisplayRectangle.Height ); 204: 205: //Fill the background image 206: e.Graphics.FillRectangle( fontProperties.BackgroundBrush, drawZone ); 207: 208: 209: 210: SizeF stringSize = e.Graphics.MeasureString( fontProperties.Text, fontProperties.Font ); 211: 212: 213: System.Drawing.Drawing2D.GraphicsState gs = e.Graphics.Save( ); 214: 215: //Center the Text 216: Point ptCenter = new Point(drawZone.X + (drawZone.Width/2), drawZone.Height / 2 ); 217: 218: //Set the Transform to the center of the drawZone 219: e.Graphics.TranslateTransform(ptCenter.X, ptCenter.Y ); 220: 221: e.Graphics.RotateTransform( fontProperties.Rotation ); 222: 223: //The Coordinate system now stems from the center of the DrawZone 224: 225: Point ptText = new Point( ); 226: ptText.X = -(int)(stringSize.Width / 2); 227: ptText.Y = -(int)(stringSize.Height / 2); 228: 229: 230: //Draw the Shadowed Text 231: int offset = fontProperties.ShadowOffset; 232: e.Graphics.DrawString( fontProperties.Text, 233: fontProperties.Font, 234: fontProperties.ShadowBrush, new Point(ptText.X + offset,ptText.Y + offset) ); 235: 236: e.Graphics.DrawString( fontProperties.Text, 237: fontProperties.Font, 238: fontProperties.FontBrush, ptText); 239: 240: 241: e.Graphics.Restore( gs ); 242: 243: } 244: /// <summary> 245: /// Clean up any resources being used. 246: /// </summary> 247: protected override void Dispose( bool disposing ) 248: { 249: if( disposing ) 250: { 251: if (components != null) 252: { 253: components.Dispose(); 254: } 255: } 256: base.Dispose( disposing ); 257: } 258: 259: #region Windows Form Designer generated code 260: /// <summary> 261: /// Required method for Designer support - do not modify 262: /// the contents of this method with the code editor. 263: /// </summary> 264: private void InitializeComponent() 265: { 266: this.splitter1 = new System.Windows.Forms.Splitter(); 267: this.propertyGrid1 = new System.Windows.Forms.PropertyGrid(); 268: this.SuspendLayout(); 269: // 270: // splitter1 271: // 272: this.splitter1.Location = new System.Drawing.Point(160, 0); 273: this.splitter1.Name = "splitter1"; 274: this.splitter1.Size = new System.Drawing.Size(3, 341); 275: this.splitter1.TabIndex = 1; 276: this.splitter1.TabStop = false; 277: this.splitter1.SplitterMoved += new System.Windows.Forms.SplitterEventHandler( this.splitter1_SplitterMoved); 278: // 279: // propertyGrid1 280: // 281: this.propertyGrid1.CommandsBackColor = System.Drawing.SystemColors.Window; 282: this.propertyGrid1.CommandsVisibleIfAvailable = true; 283: this.propertyGrid1.Dock = System.Windows.Forms.DockStyle.Left; 284: this.propertyGrid1.LargeButtons = false; 285: this.propertyGrid1.LineColor = System.Drawing.SystemColors.ScrollBar; 286: this.propertyGrid1.Name = "propertyGrid1"; 287: this.propertyGrid1.Size = new System.Drawing.Size(160, 341); 288: this.propertyGrid1.TabIndex = 0; 289: this.propertyGrid1.Text = "propertyGrid1"; 290: this.propertyGrid1.ViewBackColor = System.Drawing.SystemColors.Window; 291: this.propertyGrid1.ViewForeColor = System.Drawing.SystemColors.WindowText; 292: // 293: // Form1 294: // 295: this.AutoScaleBaseSize = new System.Drawing.Size(5, 13); 296: this.BackColor = System.Drawing.SystemColors.Window; 297: this.ClientSize = new System.Drawing.Size(528, 341); 298: this.Controls.AddRange(new System.Windows.Forms.Control[] { 299: this.splitter1, 300: this.propertyGrid1} ); 301: this.Name = "Form1"; 302: this.Text = "Font Demo"; 303: this.ResumeLayout(false); 304: 305: } 306: #endregion 307: 308: /// <summary> 309: /// The main entry point for the application. 310: /// </summary> 311: [STAThread] 312: static void Main() 313: { 314: Application.Run(new Form1()); 315: } 316: 317: private void splitter1_SplitterMoved(object sender, System.Windows.Forms.SplitterEventArgs e) { 318: this.Invalidate( ); 319: } 320: } 321: } The Font Demo application makes use of the System.ComponentModel.Browsable attribute. This attribute is used by the property grid; it determines whether the property will be available with the property grid. Expect to make use of this attribute to specify properties that are not appropriate for design-time editing. The Font Demo uses the same basic application shell as the Pen Demo. The property grid is used to manipulate the various properties of the current font object, and those properties determine how the font will appear when rendered. |