For the most part, the form validation I've demonstrated has been rather minimal, often just checking if a variable has any value at all. In many situations, this really is the best you can do. For example, there's no clear pattern for what a valid street address is or what a user might enter into a comments field. Still, much of the data you'll work with can be validated in stricter ways. Later in the chapter, the sophisticated concept of regular expressions will demonstrate just that. But here I'll cover the more approachable ways you can validate some data by type. PHP supports many types of data: strings, numbers (integers and floats), arrays, and so on. For each of these, there's a specific function that checks if a variable is of that type (Table 10.1). You've probably already seen the is_numeric() function in action in earlier chapters, and is_array() is a great for confirming a variable's type before attempting to use it in a foreach loop. Table 10.1. These functions return trUE if the submitted variable is of a certain type and FALSE otherwise.Type Validation Functions |
---|
FUNCTION | CHECKS FOR |
---|
Is_array() | Arrays | Is_bool() | Booleans (TRUE, FALSE) | Is_float() | Floating-point numbers | Is_int() | Integers | Is_null() | NULLs | Is_numeric() | Numeric values, even as a string (e.g., "20") | Is_resource() | Resources, like a database connection | Is_scalar() | Scalar (single-valued) variables | Is_string() | Strings |
In PHP, you can even change a variable's type, after it's been assigned a value. Doing so is called type casting and is accomplished by preceding a variable's name by the type in parentheses: $var = 20.2; echo (int) $var; // 20 Depending upon the original and destination types, PHP will convert the variable's value accordingly: $var = 20; echo (float) $var; // 20.0 With numeric values, the conversion is straightforward, but with other variable types, more complex rules apply: $var = 'trout'; echo (int) $var; // 0 In most circumstances you don't need to cast a variable from one type to another, as PHP will often automatically do so as needed. But forcibly casting a variable's type can be a good security measure in your Web applications. To show how you might use this notion, I'll create a calculator script for determining the total purchase price of an item, similar to that defined in earlier chapters. To use type casting 1. | Begin a new PHP document in your text editor or IDE (Script 10.5).
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-transitional.dtd> <html xmlns="http://www.w3.org/1999/ xhtml xml:lang="en" lang="en"> <head> <meta http-equiv="content-type" content="text/html; charset= iso-8859-1 /> <title>Widget Cost Calculator </title> </head> <body> <?php # Script 10.5 - calculator.php Script 10.5. By type-casting variables, this script more definitively validates that data is of the correct format. 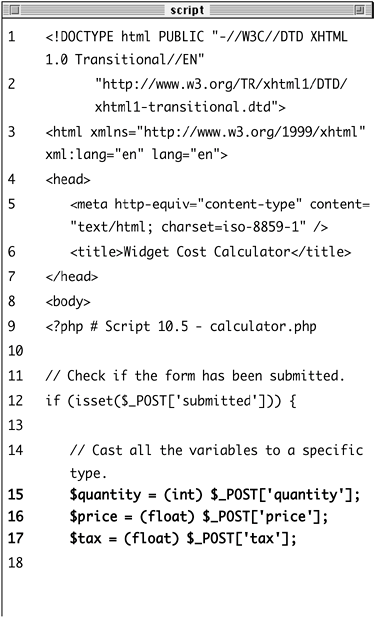 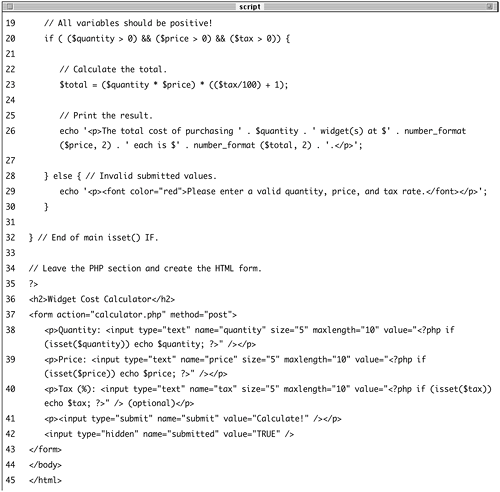
| 2. | Check if the form has been submitted.
if (isset($_POST['submitted'])) { Like many previous examples, this one script will both display the HTML form and handle its submission. By checking for the presence of a specific $_POST element, I'll know if the form has been submitted.
| 3. | Cast all the variables to a specific type.
$quantity = (int) $_POST ['quantity]; $price = (float) $_POST['price']; $tax = (float) $_POST['tax']; The form itself has three text boxes (Figure 10.11), into which practically anything could be typed (there's no number type of input for HTML forms). But I know that the quantity must be an integer and that both price and tax should be floats (contain decimal points). To force the issue, I'll cast each one to a specific type.
Figure 10.11. The HTML form takes three inputs: a quantity, a price, and a tax rate. 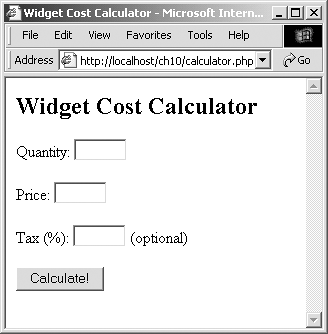
| 4. | Check if the variables have proper values, and then calculate and print the results.
if ( ($quantity > 0) && ($price > 0) && ($tax > 0)) { $total = ($quantity * $price) * (($tax/100) + 1); echo '<p>The total cost of purchasing ' . $quantity . ' widget(s) at $' . number_format ($price, 2) . ' each is $' . number_format ($total, 2) . '.</p>'; Besides knowing what type each variable should be, I also know that they must all be positive numbers. This conditional checks for that prior to performing the calculations.
The calculation itself and the printing of the results proceed much as in previous examples of the calculator. Essentially the quantity is multiplied by the price. This is then multiplied by the tax divided by 100 (so 8% becomes .08) plus 1 (1.08). The number_format() function is used to print both the price and total values in the proper format.
| 5. | Complete the conditionals.
} else { echo '<p><font color="red"> Please enter a valid quantity, price, and tax rate.</font> </p>'; } }
| 6. | Create the HTML form.
?> <h2>Widget Cost Calculator</h2> <form action="calculator.php" method="post> <p>Quantity: <input type="text" name="quantity size="5" maxlength="10" value="<?php if (isset($quantity)) echo $quantity; ?>" /></p> <p>Price: <input type="text" name="price size="5" maxlength="10 value="<?php if (isset($price)) echo $price; ?>" /></p> <p>Tax (%): <input type="text" name="tax size="5" maxlength="10 value="<?php if (isset($tax)) echo $tax; ?>" /> (optional)</p> <p><input type="submit" name="submit value="Calculate!" /></p> <input type="hidden" name="submitted value="TRUE" /> </form> The HTML form is fairly simple. I did give the inputs a sticky quality, so the user can see what was previously entered. By referring to $quantity etc. instead of $_POST['quantity'] etc., the form will reflect the value for each input as it was type-casted.
| 7. | Complete the HTML code.
</body> </html>
| 8. | Save the file as calculator.php, upload it to your Web server, and test in your Web browser (Figures 10.12 and 10.13).
Figure 10.12. If invalid values are entered, such as floats for the quantity or strings for the price… 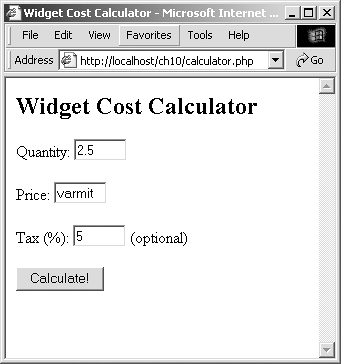
Figure 10.13. …they'll be cast into more appropriate formats. 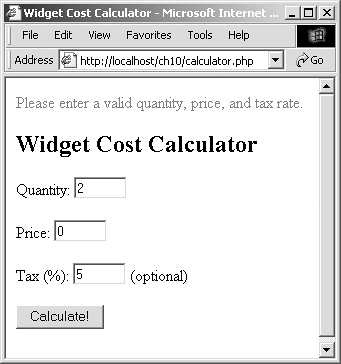
| Tips You should definitely use type casting when working with numbers within SQL queries. Numbers aren't quoted in queries, so if a string is somehow used in a number's place, there will be a SQL syntax error. If you type-cast such variables to an integer or float first, the query may not work (in terms of returning a record) but will still be syntactically valid. You'll frequently see this in the book's last three chapters. As I implied, regular expressions are a more advanced method of data validation and are sometimes your best bet. But using type-based validation, when feasible, will certainly be faster (in terms of processor speed) and less prone to programmer error (did I mention that regular expressions are complex?). To repeat myself, the rules of how values are converted from one data type to another are somewhat complex. If you want to get into the details, see the PHP manual. The gettype() function returns the type of a submitted variable.
|