Another option for error management with your sites (aside from adjusting the error reporting) is to alter how PHP handles errors. You can create your own functions that will overrule PHP's default behavior (printing them out in HTML tags). For example, function report_errors ($num, $msg) { // Do whatever here. } set_error_handler ('report_errors'); The set_error_handler() determines what function handles errors. Feeding this function the name of another function will result in that second function (e.g., report_errors) being called whenever an error is encountered. This function (report_errors) will, at that time, receive two argumentsan error number and a textual error messagewhich can be used in any possible manner. This function can also be written to take up to five arguments, the extra three being the name of the file where the error was found, the specific line number, and the variables that existed at the time of the error. function report_errors ($num, $msg, $file, $line, $vars) {... This next file will define an error handler, which will then be included in a second file that purposefully causes a couple of errors (in order to test the system). To create your own error handler 1. | Begin a new PHP script in your text editor or IDE (Script 6.2).
<?php # Script 6.2 - handle.inc.php Script 6.2. A special function is created to handle any errors that occur in a more customized manner. 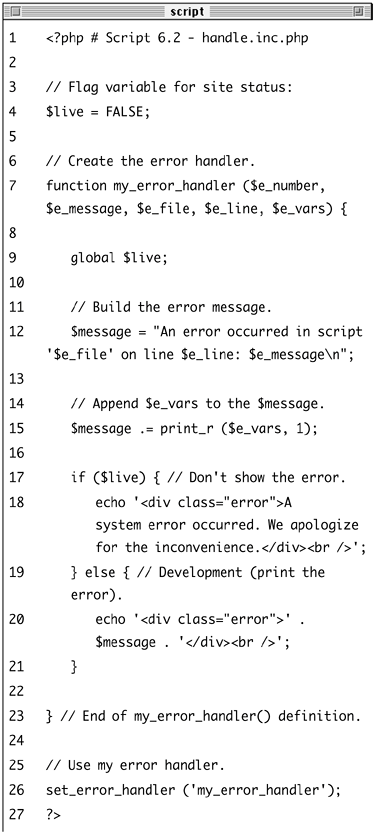
| 2. | Create a flag variable.
$live = FALSE; This variable will be a flag used to indicate whether or not the site is currently live. It's an important distinction, as how you handle errors and what you reveal in the browser should differ greatly when you're developing a site and when a site is live.
| 3. | Begin defining the error handling function.
function my_error_handler ($e_number, $e_message, $e_file, $e_line, $e_vars) { The my_error_handler() function is set to receive the full five arguments that a custom error handler can.
| 4. | Make the $live variable global.
global $live; This variable, defined in Step 2, is the flag indicating what stage the site is in (development or live). To access the variable, the global keyword must be used here. See the section on variable scope in Chapter 3, "Creating Dynamic Web Sites," for more.
| 5. | Create the error message using the received values.
$message = "An error occurred in script '$e_file on line $e_line: $e_message\n; The error message will begin by referencing the filename and number where the error occurred. Added to this is the actual error message.
| 6. | Add any existing variables to the error message.
$message .= print_r ($e_vars, 1); The $e_vars variable will receive all of the variables that exist, and their values, when the error happens. Because this might contain useful debugging information, I add it to the message.
The print_r() function is normally used to print out a variable's structure and value; it is particularly useful with arrays. If you call the function using the second argument (1), the result is returned instead of printed. So this line adds all of the variable information to $message.
| 7. | Print a message that will vary, depending upon whether or not the site is live.
if ($live) { echo '<div >A system error occurred. We apologize for the inconvenience.</div> <br />'; } else { echo '<div >' . $message . '</div><br />'; } If the site is live, a simple mea culpa will be printed, letting the user know that an error occurred but not what the specific problem is. If $live is false (when the site is being developed), the full message is printed instead.
| 8. | Complete the function and tell PHP to use it.
} set_error_handler ('my_error_ handler); This second line is the important one, telling PHP to use the custom error handler instead of PHP's default handler.
| 9. | Finish the PHP page.
?>
| 10. | Save the file as handle.inc.php and upload it to your Web server.
| To test the error handler, I'll create a dummy file that uses it to handle two silly mistakes. To create errors 1. | Begin a new PHP document in your text editor, starting with the HTML head (Script 6.3).
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-transitional.dtd> <html xmlns="http://www.w3.org/1999/ xhtml xml:lang="en" lang="en"> <head> <meta http-equiv="content-type" content="text/html; charset=iso-8859-1 /> <title>OOPS!</title> </head> <body> Script 6.3. This page includes the custom error handler and then makes two mistakes. 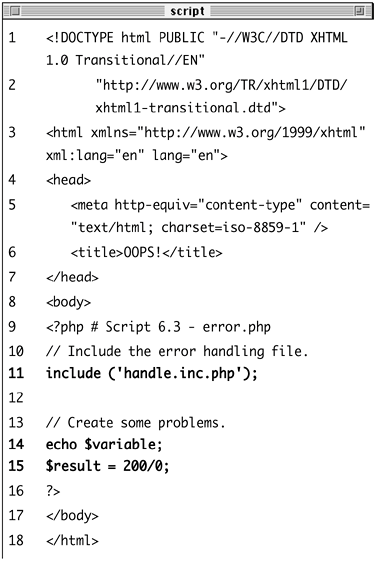
| 2. | Open a section of PHP and include the error handling file.
<?php # Script 6.3 - error.php include ('handle.inc.php'); For this, or any, script to use the custom error handler, the handle.inc.php page just needs to be included.
| 3. | Create a couple of purposeful errors to test the script.
echo $variable; $result = 200/0; In order to see how the error reporting works, two simple errors are created. The problem with the first line is that the $variable variable is referenced before it has been assigned a value (this normally causes a notice in PHP).
The second line is a classic division by zero, which is not allowed in programming languages or in math.
| 4. | Close the PHP code and the HTML page.
?> </body> </html>
| 5. | Save the file as error.php, upload it to your Web server (in the same directory as handle.inc.php), and run in your Web browser (Figure 6.9).
Figure 6.9. During the development phase, detailed error messages are printed in the Web browser. 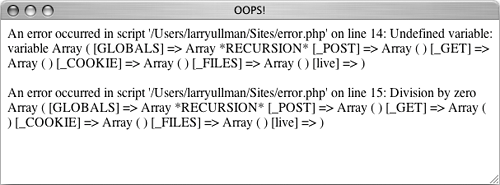
| 6. | Open handle.inc.php and change the value of $live to TRUE.
To see how the error handler behaves with a live site, just change this one value.
| 7. | Save the file, upload it to your Web server, and test again by rerunning error.php (Figure 6.10).
Figure 6.10. Once a site has gone live, more user-friendly (and less revealing) errors are printed. 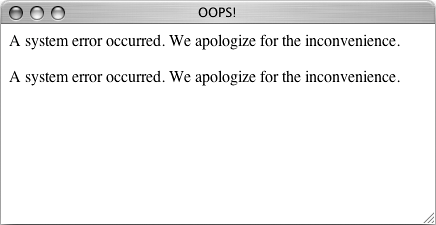
| Tips If your PHP page uses special HTML formattinglike CSS tags to affect the layout and font treatmentadd this information to your error reporting function. Obviously in a live site you'll probably need to do more than apologize for the inconvenience (particularly if a significantly affecting error occurs). Still, this example demonstrates how you can easily adjust error handling to suit the situation. You can also invoke your error handling function using trigger_error(). New to PHP 4.3 is the debug_backtrace() function, which provides a backtrace, a list of information similar to that sent to an error handler. PHP 5 takes this one step further with debug_print_backtrace(), which prints out the backtrace data.
|