The last language construct I'll discuss is loops. You've already used one, foreach, when managing arrays. The next two types of loops you'll use are for and while. The while loop looks like this: while (condition) { // Do something. } As long as the condition part of the loop is true, the loop will be executed. Once it becomes false, the loop is stopped (Figure 2.23). If the condition is never true, the loop will never be executed. The while loop will most frequently be used when retrieving results from a database, as you'll see in Chapter 7, "Using PHP with MySQL." Figure 2.23. A flowchart representation of how PHP handles a while loop. 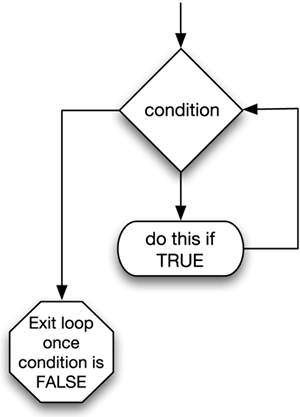
The for loop has a more complicated syntax: for (initial expression; condition; Upon first executing the loop, the initial expression is run. Then the condition is checked and, if true, the contents of the loop are executed. After execution, the closing expression is run and the condition is checked again. This process continues until the condition is false (Figure 2.24). As an example, for ($i = 1; $i <= 10; $i++) { echo $i; } Figure 2.24. A flowchart representation of how PHP handles the more complex for loop. 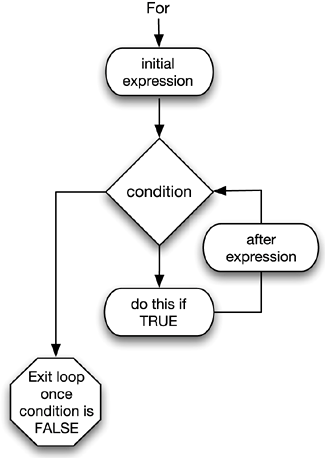
The first time this loop is run, the $i variable is set to the value of 1. Then the condition is checked (is 1 less than or equal to 10?). Since this is true, 1 is printed out (echo $i). Then, $i is incremented to 2 ($i++), the condition is checked, and so forth. The result of this script will be the numbers 1 through 10 printed out. In this chapter's last example, the calendar script created earlier will be rewritten using while and for loops in place of foreach loops. To use loops 1. | Open calendar.php (refer to Script 2.7) in your text editor.
| 2. | Delete the creation of the $days and $years arrays (lines 1718).
Using loops, I can achieve the same result of the two pull-down menus without the extra code and memory overhead involved with an array.
| 3. | Rewrite the $days foreach loop as a for loop (Script 2.12).
for ($day = 1; $day <= 31; $day++) { echo "<option value=\"$day\"> $day</option>\n; } Script 2.12. Loops are often used in conjunction with or in lieu of an array. 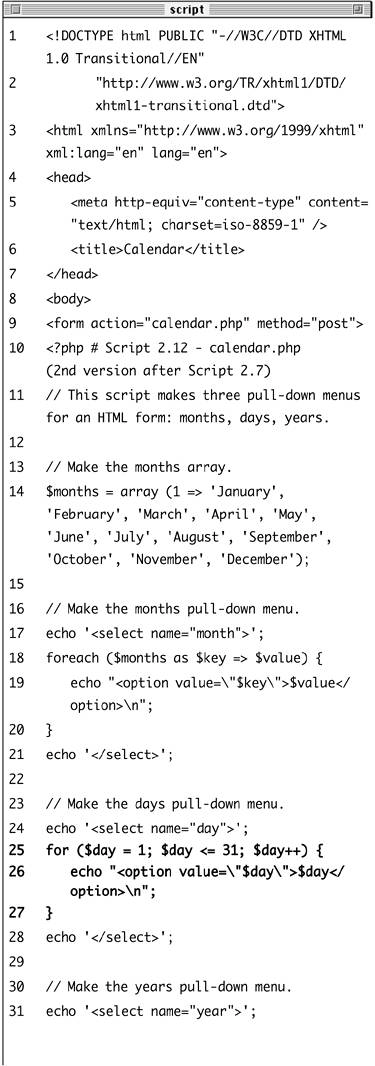 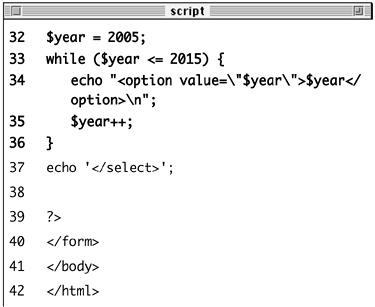
This standard for loop begins by initializing the $day variable as 1. It will continue the loop until $day is greater than 31, and upon each iteration, $day will be incremented by 1. The content of the loop itself (which is executed 31 times) is an echo() statement.
| 4. | Rewrite the $years foreach loop as a while loop.
$year = 2005; while ($year <= 2015) { echo "<option value=\"$year\"> $year</option>\n; $year++; } The structure of this loop is fundamentally the same as the previous for loop, but rewritten as a while. The $year variable is initially set, and as long as it is less than or equal to 2015, the loop will be executed. Within the loop, the echo() statement is run and then the $year variable is incremented.
| 5. | Save the file, upload to your Web server, and test in your Web browser (Figure 2.25).
Figure 2.25. The calendar form looks quite the same as it had previously (see Figure 2.16) but was created with two fewer arrays (refer to Script 2.12). 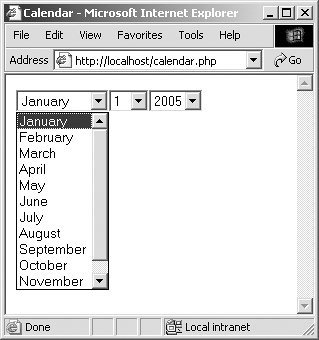
| Tips PHP also has a do...while loop with a slightly different syntax (check the manual). This loop will always be executed at least once. The syntax and functionality are similar enough that the for and while loops can often be used interchangeably as I did here. Still, experience will reveal when the for loop is a better choice (when doing something a set number of times) versus when you'd use while (doing something as long as a condition is true). When using loops, watch your parameters and conditions to avoid the dreaded infinite loop, which occurs when the loop's condition is never going to be false.
|