In Chapter 12, I showed you how to use evt to find the object in which an event originated. Using the browser's DOM, though, you can also determine the ID of the object in which the event occurred. For Internet Explorer, this entails querying the srcElement object; for W3C-compliant browsers, it means using the target object (Figures 14.1 and 14.2). Figure 14.1. The general syntax for using an event object to find the triggering ID in Internet Explorer. 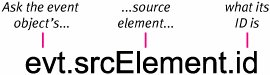 Figure 14.2. The general syntax for using an event object to find the triggering ID in W3C-compliant browsers. 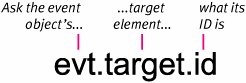 In this example, (Figure 14.3), clicking any of the four objects will cause an alert to appear, displaying the ID value for the clicked element. Figure 14.3. Pick an Alice, any Alice. To determine the element in which the event occurred: | | 1. | function findObjectID(evt) {...} Add the function findObjectID() to the JavaScript in the head of your document (Code 14.1). This script determines the CSS element on the screen in which the event occurred and then displays an alert telling you which one it was. To do this, we'll need to adapt the event equalizer to find the target (W3C browsers) or source element (Internet Explorer) of the event, which can then be used to find the object's ID:
var objectID = (evt.target) ? evt.target.id : ((evt.srcElement) ? evt.srcElement.id : null); Code 14.1. The findObjectID() function will identify the object that triggered the event by using the evt object that is passed to it. [View full width] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD /xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>CSS, DHTML & Ajax | Detecting which Object was Clicked</title> <script type="text/javascript"> function findObjectID(evt) { var objectID = (evt.target) ? evt.target.id : ((evt.srcElement) ? evt.srcElement.id : null); if (objectID) { alert('You clicked ' + objectID + '.'); } return; } </script> <style type="text/css" media="screen"> .dropBox { text-align: right; padding: 4px; background-color: #FFFFFF; border: 2px solid #f00; cursor: pointer; } #object1 { position: absolute; z-index: 3; top: 285px; left: 315px; background: white url(alice22a.gif) no-repeat; height:220px; width:150px; } #object2 { position: absolute; z-index: 2; top: 210px; left: 210px; background: white url(alice15a.gif) no-repeat; height:264px; width:200px; } #object3 { position: absolute; z-index: 1; top: 105px; left: 105px; background: white url(alice28a.gif) no-repeat; height:336px; width:250px;} #object4 { position: absolute; z-index: 0; top: 5px; left: 5px; background: white url(alice30a.gif) no-repeat; height:408px; width:300px; } </style> </head> <body> <div onclick="findObjectID(event)"> Object 1</div> <div onclick="findObjectID(event)"> Object 2</div> <div onclick="findObjectID(event)"> Object 3</div> <div onclick="findObjectID(event)"> Object 4</div> </body></html> | | 2. | #object1 {...} Set up your CSS rules, using whatever style properties you want. I set up four images (object1, object2, object3, and alice4), each with a unique ID.
| 3. | onclick="findObjectID(event);" Add an event handler to trigger the function you created in Step 1, and pass to it the event object.
| Tip Once the object ID has been found using the evt variable, you can use that information to address the object to find its properties, as explained in the next section, or make dynamic changes, as described in Chapters 16 through 18. |