You can add text fields programmatically with the createTextField() method. Like the attachMovie() and createEmptyMovieClip() methods, the createTextField() method is a MovieClip method, and it adds the new instance nested within the object from which the method is called. The createTextField() method requires six parameters: instanceName: The instance name of the new text field depth: The depth of the new text field x: The x coordinate y: The y coordinate width: The width of the new text field height: The height of the new text field The following code adds a new text field called exampleField: this.createTextField("exampleField",¬ this.getNextHighestDepth(), 0, 0, 100, 25); Like attachMovie() and createEmptyMovieClip(), the createTextField() method returns a reference to the new object. When you add text fields you don't necessarily always know the dimensions before you apply the text. However, you always have to specify some value for the width and height properties of the createTextField() method. In the event that you want the text field to have the same dimensions of the text, you can use the autoSize property with the text field and set the initial dimensions to 0. The following example illustrates how that works: var exampleField:TextField = this.createTextField("exampleField",¬ this.getNextHighestDepth(), 0, 0, 0, 0); exampleField.autoSize = "left"; exampleField.text = "Example Text"; In the following task you'll add hints to the memory game. 1. | Open the Flash document you completed in the previous task. Optionally, you can open memory4.fla from the Lesson08/Completed directory.
The Flash document contains all the necessary assets and code as the starting point for this task.
| 2. | Select the first keyframe of the main Timeline and open the Actions panel. Then add the following code following the variable declaration for correct:
var hints:Object = new Object(); hints.A = "explosion"; hints.B = "iron"; hints.C = "buttons"; hints.D = "text"; hints.E = "building"; hints.F = "fire"; var currentHint:MovieClip; var hintInterval:Number; The hints object is an associative array that stores the hint text for each of the movie clips using the linkage identifier as the key. The currentHint variable stores a reference to the current hints object, and the hintInterval variable stores the interval ID that adds the hint after a slight delay.
| 3. | Define onRollOver() and onRollOut() methods for the card movie clip. Add the following code within the initialize() method following the onPress() method definition:
card.onRollOver = function():Void { startHintInterval(hints[clips[this._name].id]); }; card.onRollOut = function():Void { currentHint.removeMovieClip(); clearInterval(hintInterval); }; When the user moves the mouse over a card, call the startHintInterval() function (defined in the next step.) When the user moves the mouse off of a card, remove the current hint and clear the interval set by startHintInterval().
| 4. | Define startHintInterval():
function startHintInterval(hintText:String):Void { hintInterval = setInterval(this, "addHint", 1000, hintText); } The startHintInterval() function sets an interval so that Flash calls addHint() in one second. That way there's a delay before the hint appears.
| 5. | Define addHint():
function addHint(hintText:String):Void { currentHint.removeMovieClip(); var depth:Number = this.getNextHighestDepth(); var hintClip:MovieClip = this.createEmptyMovieClip("clip" + depth, depth); var hint:TextField = hintClip.createTextField("hint",¬ hintClip.getNextHighestDepth(), 0, 0, 0, 0); hint.autoSize = "left"; hint.background = true; hint.border = true; hint.text = hintText; hint.selectable = false; hintClip._x = _xmouse; hintClip._y = _ymouse - hint._height; currentHint = hintClip; } The addHint() function adds a new empty movie clip that contains a text field. Then it sets some properties of the text field and moves the movie clip to the location of the mouse.
| 6. | Test the movie.
When you move the mouse over a card and pause for a second, a hint will appear.
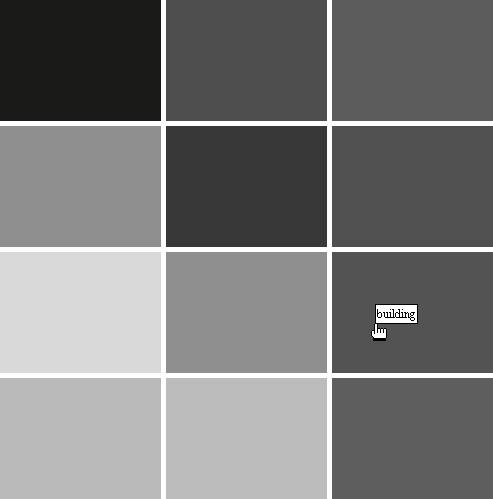 | |