The MovieClip class defines a set of methods for drawing programmatically. The set of methods are frequently called the Drawing API. The basic methods are fairly simple and they enable you to set a line style, draw line segments, draw curves, and apply fills. Each movie clip has its own line style. By default the line style is undefined, so before you can draw anything you must set the line style by way of the lineStyle() method, which requires at least one parameter specifying the line thickness in pixels. A value of 0 means that the line style is a hairline. A value of 1 or greater means that the line style has a thickness of the specified number of pixels. The maximum thickness of a line style is 255. The following code tells Flash to use a hairline for exampleClip: exampleClip.lineStyle(0); In addition to the one required parameter for lineStyle() you can also specify parameters for the following: color: A number to use for the color. The default is 0x000000 (black). alpha: A number to use for the alpha. The default is 100. pixelHinting: By default no pixelHinting is applied. If a value of true is specified, Flash snaps points to whole pixels. noScale: A string specifying how the line thickness scales when the movie clip scales. The default is normal, which means that the line scales. A value of none means that the line doesn't scale. A value of vertical means the line scales only when the movie clip is scaled vertically. A value of horizontal means the line scales only when the movie clip is scaled horizontally. capsStyle: A string specifying the line cap style. The default is round. You can also specify none and square. jointStyle: A string specifying the join style. The default is round. You can also specify miter and bevel. miterLimit: If a miter join style is applied, you can specify the miter limit. After you set a line style, you can start to draw. By default, the drawing point starts at 0,0. If you want to start drawing from a different point, you can use the moveTo() method to move the drawing point without drawing a line. The moveTo() method requires two parameters specifying the x and y coordinates to which you want to move the drawing point. exampleClip.moveTo(100, 400); The lineTo() method works just like the moveTo() method, except that it draws the line to the new point. exampleClip.lineTo(200, 200); The curveTo() method is slightly more complex because you have to specify not only the new endpoint but also the control point that determines the curve. The control point is a point that forms a tangent to the curve from both endpoints. The following code draws a curve from 100,100 to 200,100 with a control point at 150, 0. exampleClip.moveTo(100, 100); exampleClip.curveTo(150, 0, 200, 100); 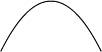 Aside from drawing lines, you can also apply fills using the beginFill() and endFill() methods. When you call the beginFill() method, Flash applies the specified fill to any closed shape that is formed by lineTo() and curveTo() methods following the method call until you call endFill(). The beginFill() method requires at least one parameter specifying the fill color. You can also specify a second parameter to control the alpha of the fill. The following code applies a blue fill to a rectangle. exampleClip.lineStyle(0); exampleClip.beginFill(0x0000FF); exampleClip.lineTo(550, 0); exampleClip.lineTo(550, 400); exampleClip.lineTo(0, 400); exampleClip.lineTo(0, 0); exampleClip.endFill(); The next task uses the Drawing API to draw movie clips corresponding to each of the movie clips attached in the preceding task. 1. | Open the Flash document you completed in the previous task. Optionally, you can open memory2.fla from the Lesson08/Completed directory.
The Flash document has all the elements to build this stage of the application.
| 2. | Select the first keyframe of the main Timeline and open the Actions panel. Then add the following code:
var clips:Object = new Object(); The clips object is an associative array that keeps track of which drawn movie clips correspond to which image movie clips.
| 3. | Within the initialize() function add the following code just after declaring the clip variable:
var card:MovieClip; The card variable is used to store a reference to the new movie clips.
| 4. | Next, within the for statement, just after attaching the movie clip instance, add the following code.
card = drawRectangle(Math.random() * 0xFFFFFF, clip._width, ¬ clip._height, x, y); clips[card._name] = {id: linkageArr[i], clip: clip}; The drawRectangle() function is a custom function defined in the next step. It adds a new movie clip and it draws a rectangle in the new movie clip. Then, use the card movie clip's instance name as the key for the clips associative array and assign to that element an object that contains the corresponding linkage identifier of the image movie clip and a reference to the image movie clip. That way, you can retrieve the corresponding image movie clip and linkage identifier when the user clicks on the rectangle card clip.
| 5. | Define the drawRectangle() function.
function drawRectangle(color:Number, width:Number, ¬ height:Number, x:Number, y:Number):MovieClip { var depth:Number = this.getNextHighestDepth(); var clip:MovieClip; clip = this.createEmptyMovieClip("clip" + depth, depth); clip.lineStyle(0, 0, 0); clip.beginFill(color); clip.lineTo(width, 0); clip.lineTo(width, height); clip.lineTo(0, height); clip.lineTo(0, 0); clip.endFill(); clip._x = x; clip._y = y; return clip; } The drawRectangle() function simply adds a new empty movie clip and then it draws a rectangle and returns a reference to the new object.
| 6. | Test the movie.
When you test the movie you'll see randomly colored rectangles in front of each of the image movie clips.
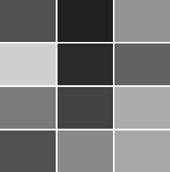 | |