14.9. Example: A Navigation Bar in a Frame This chapter ends with an example that demonstrates many of the more important window scripting techniques described here: Querying the current URL with location.href and loading new documents by setting the location property Using the back( ) and forward( ) methods of the History object Using setTimeout( ) to defer the invocation of a function Opening a new browser window with window.open( ) Using JavaScript in one frame to interact with another frame Coping with the restrictions imposed by the same-origin policy Example 14-7 is a script and simple HTML form designed to be used in a framed document. It creates a simple navigation bar in one frame and uses that bar to control the content of another frame. The navigation bar includes a Back button, a Forward button, and a text field in which the user can type a URL. You can see the navigation bar near the bottom of Figure 14-4. Figure 14-4. A navigation bar 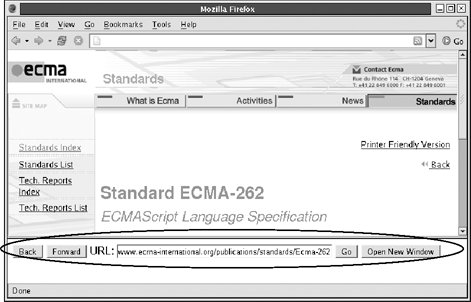 Example 14-7 defines JavaScript functions in a <script> and buttons and a URL-entry text field in a <form> tag. It uses HTML event handlers to invoke functions when the buttons are clicked. I haven't yet discussed event handlers or HTML forms in detail, but these aspects of the code are not important here. In this example, you'll find uses of the Location and History objects, the setTimeout( ) function, and the Window.open( ) function. You'll see how the JavaScript code in the navigation bar frame refers to the other frame by name and also see the use of try/catch blocks in places where the same-origin policy may cause the code to fail. Example 14-7. A navigation bar <!-- This file implements a navigation bar, designed to go in a frame. Include it in a frameset like the following: <frameset rows="*,75"> <frame src="/books/2/427/1/html/2/about:blank" name="main"> <frame src="/books/2/427/1/html/2/navigation.html"> </frameset> The code in this file will control the contents of the frame named "main" --> <script> // The function is invoked by the Back button in our navigation bar function back( ) { // First, clear the URL entry field in our form document.navbar.url.value = ""; // Then use the History object of the main frame to go back // Unless the same-origin policy thwarts us try { parent.main.history.back( ); } catch(e) { alert("Same-origin policy blocks History.back( ): " + e.message); } // Display the URL of the document we just went back to, if we can. // We have to defer this call to updateURL( ) to allow it to work. setTimeout(updateURL, 1000); } // This function is invoked by the Forward button in the navigation bar. function forward( ) { document.navbar.url.value = ""; try { parent.main.history.forward( ); } catch(e) { alert("Same-origin policy blocks History.forward( ): "+e.message);} setTimeout(updateURL, 1000); } // This private function is used by back( ) and forward( ) to update the URL // text field in the form. Usually the same-origin policy prevents us from // querying the location property of the main frame, however. function updateURL( ) { try { document.navbar.url.value = parent.main.location.href; } catch(e) { document.navbar.url.value = "<Same-origin policy prevents URL access>"; } } // Utility function: if the url does not begin with "http://", add it. function fixup(url) { if (url.substring(0,7) != "http://") url = "http://" + url; return url; } // This function is invoked by the Go button in the navigation bar and also // when the user submits the form function go( ) { // And load the specified URL into the main frame. parent.main.location = fixup(document.navbar.url.value); } // Open a new window and display the URL specified by the user in it function displayInNewWindow( ) { // We're opening a regular, unnamed, full-featured window, so we just // need to specify the URL argument. Once this window is opened, our // navigation bar will not have any control over it. window.open(fixup(document.navbar.url.value)); } </script> <!-- Here's the form, with event handlers that invoke the functions above --> <form name="navbar" onsubmit="go( ); return false;"> <input type="button" value="Back" onclick="back( );"> <input type="button" value="Forward" onclick="forward( );"> URL: <input type="text" name="url" size="50"> <input type="button" value="Go" onclick="go( );"> <input type="button" value="Open New Window" onclick="displayInNewWindow( );"> </form> | |