14.6. Adding Keywords The KeywordService is used to ad keywords to an AdGroup and works in what should by now be a familiar fashion: The client object is created and authenticated. The keywords are obtained and wrapped in XML. The entire parameter string is wrapped in XML. A request is made to the KeywordService to add the parameterized string of keywords, using the AdGroup ID. 14.6.1. Creating a Client Object and Setting Headers The WSDL file is used to create a new SOAP client object for the KeywordService web service: $keywordwsdl = "https://adwords.google.com/api/adwords/v2/KeywordService?wsdl"; $keywordclient = new soapclient($keywordwsdl, 'wsdl'); The authentication header that was constructed and saved via session tracking is used to set the header for the service: $keywordclient->setHeaders($header); 14.6.2. Stringing Together the Keywords Effective keyword targeting is the essence of getting the most out of a CPC marketing program like AdWords. In the real world, keywords might be autogenerated using internal data. Sophisticated organizations can probably come up with proprietary algorithms based on their own historical sales and conversion information to help determine cost-efficient keywords and phrases. To keep things simple in this example, a keyword list, consisting of one or more keywords, is constructed based on the user input of keywords (an input form for the keywords is shown back in Figure 14-4).  | Each "keyword" can actually contain multiple words. For example, "digital photography tips" can be entered as one keyword. |
|
The keywords are wrapped in XML and next put together: $keyword1 = $_POST['keyword1']; $keyword2 = $_POST['keyword2']; $keyword3 = $_POST['keyword3']; $keyword1 = "<newKeywords><text>$keyword1</text><type>Broad</type></newKeywords>"; $keyword2 = "<newKeywords><text>$keyword2</text><type>Broad</type></newKeywords>"; $keyword3 = "<newKeywords><text>$keyword3</text><type>Broad</type></newKeywords>"; $keywordlist = "$keyword1 $keyword2 $keyword3";  | Each keyword has a type attribute. The possible values for this attribute are Broad, Phrase, and Exact (see Chapter 10 for more information about these matches). |
|
14.6.3. Making the Request Once the keyword list has been constructed, the AdGroup ID (retrieved using PHP session tracking) is used to make the request (along with the XML-wrapped parameter list): $keywordparamsxml = "<addKeywordList> $adgroupid $keywordlist </addKeywordList>"; $keywordarray = $keywordclient->call("addKeywordList", $keywordparamsxml); $keywordarray = $keywordarray['addKeywordList']; First check to see if this causes an error: if($keywordclient->fault) { showErrors($keywordclient); } If adding the keyword list doesn't cause a SOAP fault, then all the operations have been successful and a message to that effect can be displayed (Figure 14-5). Figure 14-5. The program has successfully created an ad, and associated keywords with it, using the AdWords API 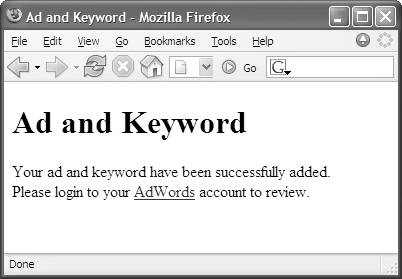 Example 14-5 shows the complete code for adding creatives and keywords to an AdGroup. Example 14-5. Creating the ad and related keywords (create_ad.php) <?php session_start( ) ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Ad and Keyword</title> </head> <body> <?php require_once('NuSOAP/nusoap.php'); require_once('hd_lib.inc'); echo "<h1>Ad and Keyword</h1>"; // Connect to the WSDL file for the CreativeService $creativewsdl = "https://adwords.google.com/api/adwords/v2/CreativeService?wsdl"; $creativeclient = new soapclient($creativewsdl, 'wsdl'); $creativeclient->setHeaders($header); $headline = $_POST['headline']; $description1 = $_POST['description1']; $description2 = $_POST['description2']; $destinationUrl = $_POST['destinationUrl']; $displayUrl = $_POST['displayUrl']; $headline = makeDocLit ("headline", $headline); $description1 = makeDocLit ("description1", $description1); $description2 = makeDocLit ("description2", $description2); $destinationUrl = makeDocLit ("destinationUrl", $destinationUrl); $displayUrl = makeDocLit ("displayUrl", $displayUrl); $creative1 = "<creative>$adgroupid $headline $description1 $description2 $destinationUrl $displayUrl </creative>"; $creativeparamsxml = "<addCreative> $creative1 </addCreative>"; $creativesarray = $creativeclient->call("addCreative", $creativeparamsxml); $creativesarray = $creativesarray['addCreativeReturn']; // Handle any SOAP errors if($creativeclient->fault) { showErrors($creativeclient); echo '<a href="authenticate.php">Try again</a>'; exit; } // The creative was created // Connect to the WSDL for the KeywordService $keywordwsdl = "https://adwords.google.com/api/adwords/v2/KeywordService?wsdl"; $keywordclient = new soapclient($keywordwsdl, 'wsdl'); $keywordclient->setHeaders($header); $keyword1 = $_POST['keyword1']; $keyword2 = $_POST['keyword2']; $keyword3 = $_POST['keyword3']; $keyword1 = "<newKeywords><text>$keyword1</text><type>Broad</type></newKeywords>"; $keyword2 = "<newKeywords><text>$keyword2</text><type>Broad</type></newKeywords>"; $keyword3 = "<newKeywords><text>$keyword3</text><type>Broad</type></newKeywords>"; $keywordlist = "$keyword1 $keyword2 $keyword3"; $keywordparamsxml = "<addKeywordList> $adgroupid $keywordlist </addKeywordList>"; $keywordarray = $keywordclient->call("addKeywordList", $keywordparamsxml); $keywordarray = $keywordarray['addKeywordList']; // Handle any SOAP errors if($keywordclient->fault) { showErrors($keywordclient); } else { echo 'Your ad and keyword have been successfully added.<br> Please login to your <a href="https://adwords.google.com/">AdWords</a> account to review.'; } ?> </body> </html> | |