Programming with JDBC is reasonably simple. All you have to do is include a few lines of code to establish your connection and you are ready to start executing SQL statements. Listing 19.1 shows a simple example. Listing 19.1 The JDBCConnection.java File package ch19; import java.sql.*; /** * * @author Stephen Potts * @version 1.0 */ public class JDBCConnection { /** Default Constructor */ public JDBCConnection() { } public static void main(String[] args) { try { //load the driver class Class.forName("sun.jdbc.odbc.JdbcOdbcDriver"); //Specify the ODBC data source String sourceURL = "jdbc:odbc:TicketRequest"; //get a connection to the database Connection databaseConnection = DriverManager.getConnection(sourceURL); //If we get to here, no exception was thrown System.out.println("The database connection is " + databaseConnection); }catch(ClassNotFoundException cnfe) { System.err.println(cnfe); } catch (SQLException sqle) { System.err.println(sqle); } } } The first step in using the driver is to specify which one that you want to use. In this case, we have specified the JDBC-ODBC bridge driver that ships with the JDK. This driver was chosen because it is always available. Class.forName("sun.jdbc.odbc.JdbcOdbcDriver"); The next step is to specify the name of the datasource that you want to use. When using the bridge driver, you specify JDBC as the protocol, and ODBC as the subprotocol. The TicketRequest is the name of the data source that you defined using the ODBC manager which we will show later in the chapter. String sourceURL = "jdbc:odbc:TicketRequest"; The goal of this program is to obtain an instance of the class Connection. Connection databaseConnection = DriverManager.getConnection(sourceURL); We will just print the connection, which will give us the name of the instance. If this executes without an exception, the program was successful. System.out.println("The database connection is " + databaseConnection); Before we can run this program, we have to set up an ODBC datasource. A datasource roughly corresponds to a table in a database. In our case, the DBMS is MS Access. The database is located in a file called c:\com\samspublishing\jpp\TicketRequest.mdb. You can copy this file to that directory from the Internet site for this book, www.samspublishing.com. Open the Control Panel on your Windows computer, and then the Administrative Tools folder (XP only). You will see an icon labeled Data Sources (ODBC). Double-clicking this icon will bring up the window shown in Figure 19.3. Figure 19.3. The ODBC Manager enables you to specify data sources that can be used by JDBC. 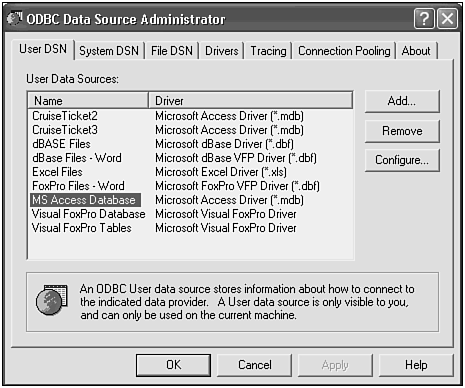 Click the Add button. It will open the window shown in Figure 19.4. Figure 19.4. You specify the type of database that the data is stored in. 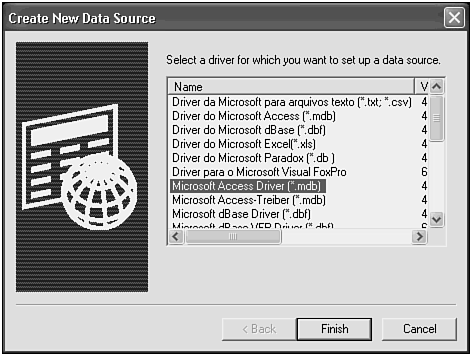 In this case, the database is MS Access, so you have to pick that driver and click the Finish button. This will open the window shown in Figure 19.5. Figure 19.5. Next, you specify the location of the database that the data is stored in. 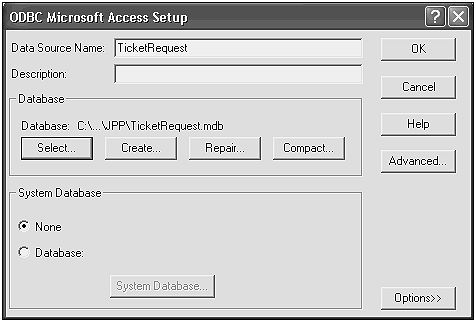 You type the name that you want the datasource to be known by in your code, and you use the browser to locate the database. On our test machine, that directory is called c:\com\samspublishing\jpp. You click the OK button, which opens the window shown in Figure 19.6. Figure 19.6. The name that you chose, TicketRequest, is now the name that the data-source is known by in your programs. 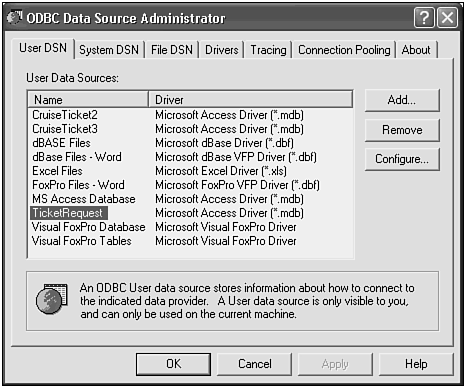 There is nothing magic about the name TicketRequest. You could have named it XYZ and it would have worked. |