One of the new filters included in Flash 8 is dropShadow. You can apply it to individual movie clips on the authoring environment's stage. You can also apply it programmatically to externally loaded images with ActionScript. This is handy because the application dynamically loads and displays external bitmap images. Applying a Filter with ActionScript Let's start with a simple example, and when you have that working, you can integrate it into the code you developed for the slide show application. 1. | Open a new Flash document and make sure that its publishing settings are set for Flash 8 and not an earlier version. On the stage, draw a shape, convert it into a movie clip, and give it an instance name of myShape_mc.
| 2. | Create an actions layer above the layer with the movie clip, select the first frame in the layer, and open the Actions panel. Next, import the class for the DropShadowFilter. At the top of the page, add the following code:
import flash.filters.DropShadowFilter; | 3. | Before you can apply the filter, you have to set up some parameters for it. The following code not only assigns values to the parameters of the filter, it does so in a way that is easy to read and modify:
// set dropshodow properties var shadowDistance:Number = 20; var shadowAngleInDegrees:Number = 45; var shadowColor:Number = 0x000000; var shadowAlpha:Number = .6; var shadowBlurX:Number = 16; var shadowBlurY:Number = 16; var shadowStrength:Number = 1; var shadowQuality:Number = 3; var shadowInner:Boolean = false; var shadowKnockout:Boolean = false; var shadowHideObject:Boolean = false; | 4. | Next, create a new DropShadowFilter object (datatype), passing the parameter values through local variables:
[View full width] var myFilter:DropShadowFilter = new DropShadowFilter(shadowDistance, shadowAngleInDegrees, shadowColor, shadowAlpha, shadowBlurX, shadowBlurY, shadowStrength, shadowQuality, shadowInner, shadowKnockout, shadowHideObject);
Although this might not seem easy to read, it is more meaningful than entering the values into the definition as it is created, which would look like this:
[View full width] var myFilter:DropShadowFilter =new DropShadowFilter (20, 45, 0x000000, .6, 16, 16, 1, 3, false, false,false);
| 5. | Now you need to create a new array to hold the filters you will apply to this movie clip. The movie clip object has an array property that holds the filters applied to it. If the array is empty, no filters are applied. This means you can apply more than one filter to a movie clip and you can specify in which order they are applied.
| 6. | Create an array for the filters applied to this movie clip and then push the filter you just created (the DropShadowFilter) into the array. Finally, save the array as the filters array of the myShape instance of the movie clip:
var filterArray:Array = new Array(); filterArray.push(myFilter); myShape.filters = filterArray; | All together, the code now looks like this: [View full width] import flash.filters.DropShadowFilter; // set dropshodow properties var shadowDistance:Number = 20; var shadowAngleInDegrees:Number = 45; var shadowColor:Number = 0x000000; var shadowAlpha:Number = .6; var shadowBlurX:Number = 16; var shadowBlurY:Number = 16; var shadowStrength:Number = 1; var shadowQuality:Number = 3; var shadowInner:Boolean = false; var shadowKnockout:Boolean = false; var shadowHideObject:Boolean = false; // create new filter object with the parameters from above var myFilter:DropShadowFilter = new DropShadowFilter(shadowDistance, shadowAngleInDegrees, shadowColor, shadowAlpha, shadowBlurX, shadowBlurY, shadowStrength, shadowQuality, shadowInner,shadowKnockout, shadowHideObject); // create array to save filters for this movie clip var filterArray:Array = new Array(); filterArray.push(myFilter); myShape.filters = filterArray;
Doublecheck that the publishing settings are set for Flash 8 and test it out. Figure 16.11 shows a black rectangle that has a DropShadowFilter applied to it by the previous code. When it's working, you can move on to integrating this into your project. Figure 16.11. Application of the new DropShadow Filter. 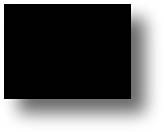 Adding a Drop Shadow to Your Slide Show Now you can incorporate this into your project by adding code to the cycleImages function. 1. | As mentioned earlier in the chapter, it's best to put the import statement at the top of your existing code to keep all import statements at the beginning of all code.
import flash.filters.DropShadowFilter; | 2. | Apply the filter to the movie clips holding the loaded images. You could put the filter code in the onloadInit function, but then the filters would be prepared before they're visible. This is generally not the best use of the end-user's system resources.
An alternative, and better option, is to put the filter code in the cycleImages function, which is called when the application is scheduled (with setInterval) to transition from one image to the next in the gallery. With the added code, the cycleImages function looks like this:
[View full width] function cycleImages(){ var iTotal = loadedImages.length;v if(control.slideNum < iTotal){ var iNext = control.slideNum; var iLast = control.slideNum - 1; } else if (control.slideNum >= iTotal){ control.slideNum = 0; var iNext = 0; var iLast = iTotal-1; } var nextImage = loadedImages[iNext]; var lastImage = loadedImages[iLast]; nextImage._visible = true; lastImage._visible = false; // set dropshodow properties var shadowDistance:Number = 20; var shadowAngleInDegrees:Number = 45; var shadowColor:Number = 0x000000; var shadowAlpha:Number = .6; var shadowBlurX:Number = 16; var shadowBlurY:Number = 16; var shadowStrength:Number = 1; var shadowQuality:Number = 3; var shadowInner:Boolean = false; var shadowKnockout:Boolean = false; var shadowHideObject:Boolean = false; var myFilter:DropShadowFilter = new DropShadowFilter(shadowDistance, shadowAngleInDegrees, shadowColor, shadowAlpha, shadowBlurX, shadowBlurY, shadowStrength, shadowQuality, shadowInner, shadowKnockout, shadowHideObject); var filterArray:Array = new Array(); filterArray.push(myFilter); nextImage.filters = filterArray; control.slideNum++; }
| 3. | To free up memory, you can also remove the filter from the filter array of the lastImage. This way, you don't have an accumulation of filters as Flash cycles through the images in the slide show:
[View full width] function cycleImages(){ var iTotal = loadedImages.length;v if(control.slideNum < iTotal){ var iNext = control.slideNum; var iLast = control.slideNum - 1; } else if (control.slideNum >= iTotal){ control.slideNum = 0; var iNext = 0; var iLast = iTotal-1; } var nextImage = loadedImages[iNext]; var lastImage = loadedImages[iLast]; nextImage._visible = true; lastImage._visible = false; // set dropshodow properties var shadowDistance:Number = 20; var shadowAngleInDegrees:Number = 45; var shadowColor:Number = 0x000000; var shadowAlpha:Number = .6; var shadowBlurX:Number = 16; var shadowBlurY:Number = 16; var shadowStrength:Number = 1; var shadowQuality:Number = 3; var shadowInner:Boolean = false; var shadowKnockout:Boolean = false; var shadowHideObject:Boolean = false; var myFilter:DropShadowFilter = new DropShadowFilter(shadowDistance, shadowAngleInDegrees, shadowColor, shadowAlpha, shadowBlurX, shadowBlurY, shadowStrength, shadowQuality, shadowInner, shadowKnockout, shadowHideObject); var filterArray:Array = new Array(); filterArray.push(myFilter); nextImage.filters = filterArray; // remove filter from image movieclip to conserve memory allocation // this is optional, but might be good to use if you have // a large collection of images lastImage.filters.pop(); control.slideNum++; }
| So, there you go: a slideshow with several additional features that make for some pretty sophisticated Flash magic. Take the code (as well as the principles behind it) and apply it to your own projects. That is the easiest way to become a really great Flash coder. |