Using the BackgroundWorker Control Using asynchronous methods on a web service is just one way in which you can speed up your application and increase your application's reliability and availability, two key factors in creating a truly agile smart client. However, your application may want to do more than just make multithreaded single calls to a web service. Quite often, smart clients need to perform background tasks and report the status of that task to the user. The BackgroundWorker component is an extremely handy tool that takes care of a lot of the tedious work that used to go into performing long-running tasks in the background and reporting progress to the foreground thread and user interface. By handling the BackgroundWorker component's three events, you can write code that executes in a background thread and you don't have to worry about the complexities of starting the thread or about using the Invoke() method to communicate with the UI thread. These events are DoWork This event handler is invoked in a background thread. Whatever method you define for this event handler is the "work" method for the component. Within this method, you check the CancellationPending property to stop execution and call the ReportProgress method to indicate the percentage of the background task that has been completed. ProgressChanged Use this event handler to respond to a change in the progress (percent complete) of the background task. This event handler is often used to modify the state of progress bars and other UI elements indicating progress. RunWorkerCompleted Use this event handler to respond to the condition when the background task has completed. To see this component in action, create a new Windows Forms application and add a StatusStrip control to the form. Using the interactive designer, you can add a progress bar to the StatusStrip control. Then you'll need a button to kick off the background process. Drag a BackgroundWorker onto the form. Next, double-click the blank spaces in the event handler boxes for all of the BackgroundWorker component's events. Listing 38.5 shows the code that makes it all work. Listing 38.5. Using the BackgroundWorker Component using System; using System.Collections.Generic; using System.ComponentModel; using System.Threading; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; namespace BgWorker { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void backgroundWorker1_DoWork(object sender, DoWorkEventArgs e) { tsProgress.Value = 0; for (int i = 0; i < 100; i++) { if (backgroundWorker1.CancellationPending) { e.Cancel = true; return; } Thread.Sleep(100); backgroundWorker1.ReportProgress(i); } } private void backgroundWorker1_ProgressChanged( object sender, ProgressChangedEventArgs e) { tsProgress.Value = e.ProgressPercentage; } private void backgroundWorker1_RunWorkerCompleted( object sender, RunWorkerCompletedEventArgs e) { if (e.Cancelled) { tsProgress.Value = 0; MessageBox.Show("Long-running task was cancelled."); } else MessageBox.Show("Long-running task completed."); } // launch background task private void button1_Click(object sender, EventArgs e) { backgroundWorker1.RunWorkerAsync(); } // cancel background task private void button2_Click(object sender, EventArgs e) { backgroundWorker1.CancelAsync(); } } } | With the task in progress, your form might look something like the one shown in Figure 38.4. Figure 38.4. Using the BackgroundWorker component. 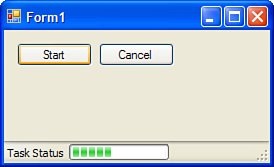 |